c语言替换字符串函数
时间: 2024-10-14 11:01:40 浏览: 27
在C语言中,标准库并没有提供专门的字符串替换函数,如Python的`str.replace()`。不过,你可以通过结合`strcpy()`、`strlen()`和指针操作来实现基本的字符串替换。下面是一个简单的例子,展示了如何替换原始字符串中的一部分内容:
```c
#include <stdio.h>
#include <string.h>
// 自定义替换字符串函数
char* replace_string(char *str, const char *old_subst, const char *new_subst) {
size_t old_length = strlen(old_subst);
size_t new_length = strlen(new_subst);
size_t index;
// 遍历整个字符串
for (index = 0; ; index++) {
// 如果找到了旧子串
if (strstr(&str[index], old_subst) != NULL) {
// 记录旧子串起始位置
size_t start_pos = index;
// 从头开始搜索新子串的位置
size_t end_pos = start_pos + old_length - 1;
while (end_pos < strlen(str) && str[end_pos] == old_subst[end_pos]) {
end_pos++;
}
// 创建一个新的临时字符串,包含旧子串之前的内容和新子串
char *temp = malloc(index + (end_pos - start_pos) + new_length + 1);
strncpy(temp, str, index);
strcat(temp, new_subst);
// 更新原字符串的这部分内容
memmove(&str[index], &str[index + old_length], end_pos - index + 1);
// 结合更新过的子串和剩余部分
strcat(str, temp + old_length);
free(temp);
// 搜索下一段旧子串
index += old_length - 1;
} else {
break; // 找不到更多旧子串就停止
}
}
return str;
}
int main() {
char str[] = "Hello, world! This is a test.";
char old[] = "world";
char new_str[] = "CSDN";
str = replace_string(str, old, new_str);
printf("替换后的字符串: %s\n", str); // 输出: Hello, CSDN! This is a test.
return 0;
}
```
这个函数会在原始字符串中查找旧子串,并将其替换为新子串。需要注意的是,这个实现仅能处理单次替换,对于连续出现的旧子串,你需要在循环里多次处理。
阅读全文
相关推荐
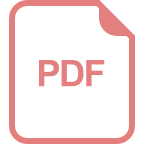
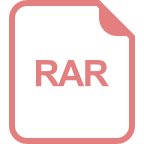

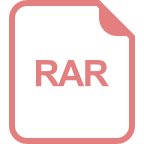
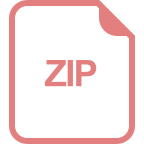
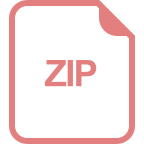


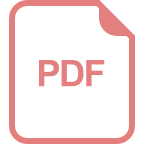
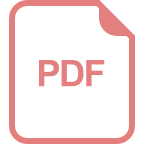
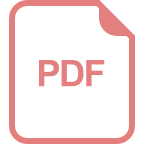
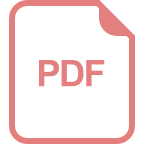
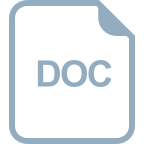
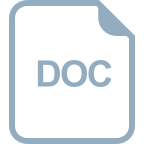
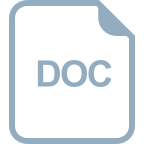


