用c#打印出几行几列
时间: 2024-10-14 22:06:23 浏览: 14
在C#中,你可以使用嵌套循环结构(通常是一个for循环嵌套另一个for循环)来打印出指定行数和列数的星号或其他字符。下面是一个简单的例子,用于打印一个二维数组(例如,星号*),其中每行有5个星号,共打印3行:
```csharp
using System;
class Program
{
static void Main(string[] args)
{
int rows = 3;
int cols = 5;
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
Console.Write("* ");
}
// 每一行结束后换行
Console.WriteLine();
}
}
}
```
当你运行这个程序,它会打印出类似这样的结果:
```
*****
*****
*****
```
相关问题
在 C# 的 DataGridView 控件中,在已经知道行列的情况下,如何获取这几行几列的参数
在 C# 的 DataGridView 控件中,如果你已经知道了特定行(行索引)和列(列名),你可以通过以下步骤来获取单元格的数据:
1. 首先,你需要创建一个 DataGridView 控件的对象,比如命名为 dataGridView。
2. 使用 `dataGridView.Rows` 属性来获取指定行索引(例如,假设你想获取第5行)的 DataGridViewRow 对象,如:
```csharp
DataGridViewRow row = dataGridView.Rows[4]; // 确保索引从0开始
```
3. 接着,使用 `DataGridViewRow.Cells` 属性来获取该行的所有列(假设你想获取“Column1”列,列名需要与实际控件中的列标题匹配):
```csharp
DataGridViewCell cell = row.Cells["Column1"];
```
4. 要获取单元格的具体值,可以使用 `.Value` 属性:
```csharp
string value = (string)cell.Value;
```
如果单元格的内容不是字符串类型,记得转换成相应的类型。
如果你需要处理动态列名或不确定列位置的情况,你还可以尝试遍历列集合并检查每一列的名称。
在 C# 的 DataGridView 控件中,在已经知道行列的情况下,如何获取这几行几列的参数,并高亮显示
在C#的DataGridView控件中,如果你想要获取特定行列的数据并高亮显示,你可以按照以下步骤操作:
1. 首先,你需要确定你要高亮的行和列的索引。假设你想高亮第`rowIndex`行和第`columnIndex`列,可以这样做:
```csharp
int rowIndex = // 你想要高亮的行的索引;
int columnIndex = // 你想要高亮的列的索引;
// 确保索引在有效范围内,避免异常
if (rowIndex >= 0 && rowIndex < dataGridView.Rows.Count &&
columnIndex >= 0 && columnIndex < dataGridView.Columns.Count)
{
DataGridViewRow row = dataGridView.Rows[rowIndex];
DataGridViewCellStyle highlightStyle = new DataGridViewCellStyle { BackColor = Color.Yellow }; // 设置高亮颜色,如黄色
// 给单元格设置高亮样式
row.Cells[columnIndex].Style = highlightStyle;
}
```
2. 如果你希望将整个行或列高亮,而不是单个单元格,可以对行或列应用`DefaultCellStyle`:
- 对于一行高亮:
```csharp
DataGridViewRowHighlighting highlightingType = DataGridViewRowHeaderMouseClick; // 指定鼠标点击事件触发高亮
dataGridView.Rows[rowIndex].DefaultCellStyle.BackColor = Color.Yellow;
dataGridView.Rows[rowIndex].DefaultCellStyle.SelectionBackColor = Color.Yellow; // 高亮背景色
dataGridView.Rows[rowIndex].Select(); // 选择该行
dataGridView.Rows[rowIndex].BeginEdit(false); // 开始编辑模式,结束时会取消高亮
dataGridView.RowHeadersDefaultCellStyle.BackColor = SystemColors.ControlDark; // 高亮行头颜色
```
- 对于一列高亮,类似地操作`Columns`集合。
完成上述操作后,目标行和列就会被高亮显示。记得处理好用户交互,例如在释放焦点或关闭编辑模式时移除高亮效果。
阅读全文
相关推荐
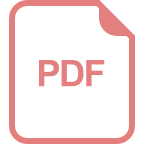
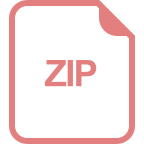
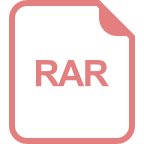
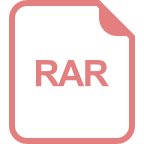
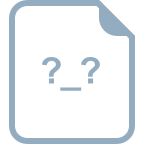
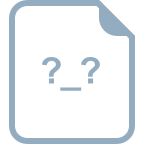
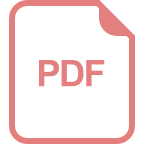
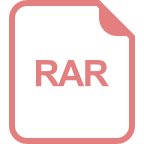
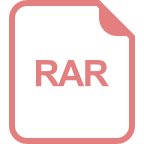
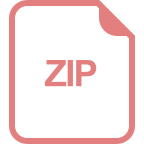
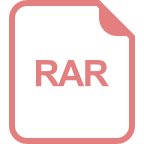
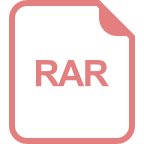
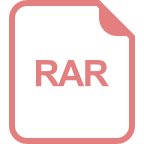



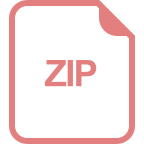