def __init__(self,data_root,data_label):
时间: 2024-01-18 07:05:05 浏览: 21
As an AI language model, I don't have access to the context of your code. But this looks like the constructor method of a class.
The `__init__` method is a special method in Python classes that is called when an object of the class is created. It initializes the object's attributes and sets them to their default values.
In this case, the method takes two parameters: `data_root` and `data_label`. These are likely the root directory and label of the data that the class will be working with. The method may use these parameters to set the initial values of some of the class's attributes or perform other initialization tasks specific to the class.
相关问题
def __init__(self, data_root, label_root, camera='kinect', split='train', input_size=(480, 480), adapt_radius=True):
根据提供的代码片段,这是一个类的构造函数`__init__`的定义。构造函数通常在创建类的实例时被调用,并用于初始化类的属性和执行其他必要的设置。
该构造函数接受以下参数:
- `data_root`:数据的根目录
- `label_root`:标签的根目录
- `camera`:相机类型,默认为'kinect'
- `split`:数据集拆分类型,默认为'train'
- `input_size`:输入图像的大小,默认为(480, 480)
- `adapt_radius`:是否使用自适应半径,默认为True
在构造函数中,你可以根据需要使用这些参数来初始化类的属性或执行其他操作。例如,你可以将传递的参数赋值给类的属性,如:
```python
self.data_root = data_root
self.label_root = label_root
self.camera = camera
self.split = split
self.input_size = input_size
self.adapt_radius = adapt_radius
```
然后,你可以在类的其他方法中使用这些属性来进行进一步的处理或操作。构造函数的具体实现取决于类的功能和需求。
pyqt5怎么显示class QueryThread(QThread): query_result = pyqtSignal(object) def __init__(self, id): super().__init__() self.id = id def run(self): conn = pymysql.connect(host='39.99.214.172', user='root', password='Solotion.123', database='jj_tset') cursor = conn.cursor() cursor.execute("SELECT * FROM employee_table WHERE user_id='%s'" % self.id) result = cursor.fetchone() conn.close() self.query_result.emit(result) # 发送查询结果信号 class MainWindow(QtWidgets.QMainWindow): def __init__(self): super().__init__() self.query_thread = None # 查询线程 def read_data(self): data = self.ser.readline() if data: id = data.decode().strip() if not self.query_thread: # 如果查询线程不存在,则创建并启动 self.query_thread = QueryThread(id) self.query_thread.query_result.connect(self.update_ui) self.query_thread.start() else: self.query_thread.id = id # 如果查询线程已存在,则更新查询ID QTimer.singleShot(100, self.read_data) def update_ui(self, result): if result: self.id_label.setText("员工ID:" + result[0]) self.name_label.setText("姓名:" + str(result[1])) self.six_label.setText("性别:" + result[2]) self.sfz_label.setText("身份证:" + str(result[3])) self.tel_label.setText("电话:" + result[4]) else: self.id_label.setText("员工ID:") self.name_label.setText("姓名:") self.six_label.setText("性别:") self.sfz_label.setText("身份证:") self.tel_label.setText("电话:") def closeEvent(self, event): self.ser.close() if self.query_thread: self.query_thread.quit() self.query_thread.wait()
要在 PyQt5 中显示这段代码,可以使用 QTextEdit 或者 QPlainTextEdit 组件。首先,需要在程序中导入相关的模块:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QTextEdit
```
然后,创建一个 QTextEdit 组件,并将代码添加到其中:
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.code_edit = QTextEdit(self)
self.code_edit.setReadOnly(True)
self.setCentralWidget(self.code_edit)
self.code_edit.setPlainText('''
class QueryThread(QThread):
query_result = pyqtSignal(object)
def __init__(self, id):
super().__init__()
self.id = id
def run(self):
conn = pymysql.connect(host='39.99.214.172', user='root', password='Solotion.123', database='jj_tset')
cursor = conn.cursor()
cursor.execute("SELECT * FROM employee_table WHERE user_id='%s'" % self.id)
result = cursor.fetchone()
conn.close()
self.query_result.emit(result) # 发送查询结果信号
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.query_thread = None # 查询线程
def read_data(self):
data = self.ser.readline()
if data:
id = data.decode().strip()
if not self.query_thread: # 如果查询线程不存在,则创建并启动
self.query_thread = QueryThread(id)
self.query_thread.query_result.connect(self.update_ui)
self.query_thread.start()
else:
self.query_thread.id = id # 如果查询线程已存在,则更新查询ID
QTimer.singleShot(100, self.read_data)
def update_ui(self, result):
if result:
self.id_label.setText("员工ID:" + result[0])
self.name_label.setText("姓名:" + str(result[1]))
self.six_label.setText("性别:" + result[2])
self.sfz_label.setText("身份证:" + str(result[3]))
self.tel_label.setText("电话:" + result[4])
else:
self.id_label.setText("员工ID:")
self.name_label.setText("姓名:")
self.six_label.setText("性别:")
self.sfz_label.setText("身份证:")
self.tel_label.setText("电话:")
def closeEvent(self, event):
self.ser.close()
if self.query_thread:
self.query_thread.quit()
self.query_thread.wait()
''')
```
这样就可以将代码显示在程序的主窗口中了。需要注意的是,这段代码中的 self.ser 没有定义,如果没有在程序中定义,会导致程序出错。
相关推荐










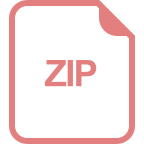