但是我已经声明过了 class satDataSet(data.Dataset): def __init__(self,root,filetypefile,transform=None): super(satDataSet,self).__init__() self.root = root self.files = [] self.transforms = transform df = pd.read_csv(filetypefile,names=['filename','satType']) for adf in df.values: dat_file = os.path.join(self.root,r"%s" % adf[0]) typename = int("%d" % adf[1]) self.files.append({ "datFile":dat_file, "typename":typename}) def __len__(self): return len(self.files) # 返回数据集的长度 def __getitem__(self, index): ##一张图像一张图像的读取,并处理,即三维的 datafiles = self.files[index] label = datafiles["typename"] #satdata = torch.from_numpy(np.load(datafiles["datFile"])["sat"]) satdata = np.load(datafiles["datFile"])["sat"] satdata = np.transpose(satdata, (1, 2, 0)) #print("satdata.shape",satdata.shape) ## 将数据纬度转化成(100, 101, 19)将通道数放在最后 # 将 NumPy 数组转换为 PIL 图像对象 #satdata = Image.fromarray(satdata) satdata = preprocess_data(satdata,opt) satdata = torch.from_numpy(satdata.transpose((2, 0, 1))) # 转换为 PyTorch 张量 label = torch.from_numpy(np.array([label])) return satdata,label
时间: 2024-04-18 16:27:32 浏览: 159
根据你提供的代码,你定义了一个名为satDataSet` 的类,该类继承自 `data.Dataset`。这是一个自定义的数据集类,用于加载和处理卫星图像数据集。
在这个自定义数据集类中,你实现了 `__init__`、`__len__` 和 `__getitem__` 方法。其中,`__init__` 方法初始化了数据集的根目录、文件列表和转换函数。`__len__` 方法返回了数据集的长度,即文件列表的长度。`__getitem__` 方法根据给定的索引,从文件列表中获取对应的文件名、标签,并进行相应的数据处理和转换操作。
这样,通过实现这些方法,你可以使用这个自定义数据集类来创建数据加载器,并在训练过程中迭代地获取样本数据。
请注意,这个自定义数据集类并不是 Python 的内置类或函数,而是你根据 `data.Dataset` 类进行的自定义实现。在使用时需要确保导入正确的模块,并按照正确的方式调用和使用该类。
相关问题
""" This code is based on the Torchvision repository, which was licensed under the BSD 3-Clause. """ import os import pickle import sys import numpy as np import torch from PIL import Image from torch.utils.data import Dataset from utils.mypath import MyPath from torchvision.datasets.utils import check_integrity, download_and_extract_archive class simclr_c10(Dataset): base_folder = 'D:/wjd/simclr_c10' filename = "simclr_c10" def __init__(self, root=MyPath.db_root_dir('wjd/simclr_c10/'), train=True, transform=None, download=False): super('wjd/simclr_c10/', self).__init__() self.data = None self.root = root self.transform = transform self.train = train # training set or test set self.classes = ['Al', 'Ag', 'Au', 'Cu', 'W', 'V', 'Ta', 'Mo'] def __getitem__(self, index): """ Args: index (int): Index Returns: dict: {'image': image, 'target': index of target class, 'meta': dict} """ img, target = self.data[index], self.targets[index] img_size = (img.shape[0], img.shape[1]) img = Image.fromarray(img) class_name = self.classes[target] if self.transform is not None: img = self.transform(img) out = {'image': img, 'target': target, 'meta': {'im_size': img_size, 'index': index, 'class_name': class_name}} return out def get_image(self, index): img = self.data[index] return img def __len__(self): return len(self.data) def extra_repr(self): return "Split: {}".format("Train" if self.train is True else "Test") return outside function,这段代码正确吗
这段代码有一些问题:
1. `super('wjd/simclr_c10/', self).__init__()` 这句话不正确,应该是 `super(simclr_c10, self).__init__()`
2. `self.targets` 在代码中没有定义,需要在 `__init__` 函数中将其定义并初始化
3. `return outside function` 这句话不应该出现在类定义中,需要将其删除
4. 另外,`check_integrity` 和 `download_and_extract_archive` 函数在代码中没有使用,可以删除
修正后的代码如下所示:
```
import os
import pickle
import sys
import numpy as np
import torch
from PIL import Image
from torch.utils.data import Dataset
from utils.mypath import MyPath
class simclr_c10(Dataset):
base_folder = 'D:/wjd/simclr_c10'
filename = "simclr_c10"
def __init__(self, root=MyPath.db_root_dir('wjd/simclr_c10/'), train=True, transform=None, download=False):
super(simclr_c10, self).__init__()
self.data = None
self.targets = []
self.root = root
self.transform = transform
self.train = train # training set or test set
self.classes = ['Al', 'Ag', 'Au', 'Cu', 'W', 'V', 'Ta', 'Mo']
self._load_data()
def _load_data(self):
if self.train:
data_file = os.path.join(self.root, self.base_folder, 'train.pkl')
else:
data_file = os.path.join(self.root, self.base_folder, 'test.pkl')
with open(data_file, 'rb') as f:
data = pickle.load(f)
self.data = data['data']
self.targets = data['targets']
def __getitem__(self, index):
"""
Args:
index (int): Index
Returns:
dict: {'image': image, 'target': index of target class, 'meta': dict}
"""
img, target = self.data[index], self.targets[index]
img_size = (img.shape[0], img.shape[1])
img = Image.fromarray(img)
class_name = self.classes[target]
if self.transform is not None:
img = self.transform(img)
out = {'image': img, 'target': target, 'meta': {'im_size': img_size, 'index': index, 'class_name': class_name}}
return out
def get_image(self, index):
img = self.data[index]
return img
def __len__(self):
return len(self.data)
def extra_repr(self):
return "Split: {}".format("Train" if self.train is True else "Test")
```
class DataFolder(data.Dataset): """ ImageFolder can be used to load images where there are no labels.""" def __init__(self, root, TreePoint,dataLenPerFile, transform=None ,loader=default_loader): # dataLenPerFile is the number of all octnodes in one 'mat' file on average dataNames = [] for filename in sorted(glob.glob(root)): if is_image_file(filename): dataNames.append('{}'.format(filename)) self.root = root self.dataNames =sorted(dataNames) self.transform = transform self.loader = loader self.index = 0 self.datalen = 0 self.dataBuffer = [] self.fileIndx = 0 self.TreePoint = TreePoint self.fileLen = len(self.dataNames) assert self.fileLen>0,'no file found!' # self.dataLenPerFile = dataLenPerFile # you can replace 'dataLenPerFile' with the certain number in the 'calcdataLenPerFile' self.dataLenPerFile = self.calcdataLenPerFile() # you can comment this line after you ran the 'calcdataLenPerFile'
这段代码定义了一个自定义的 `DataFolder` 类,该类继承自 `torchvision.datasets.Dataset` 类,用于加载图像数据集。
构造函数 `__init__` 接受以下参数:
- `root`:数据集的根目录,可以是包含图像文件的文件夹路径或包含通配符的文件路径。
- `TreePoint`:树结构的某个节点。
- `dataLenPerFile`:每个 'mat' 文件中平均包含的八叉树节点数量。
- `transform`:可选参数,用于对图像进行预处理的数据转换操作。
- `loader`:可选参数,用于加载图像的函数,默认为 `default_loader` 函数。
在构造函数中,首先通过 `glob.glob(root)` 使用通配符获取匹配 `root` 路径下的文件名列表,并使用 `is_image_file()` 函数过滤出图像文件,将它们添加到 `dataNames` 列表中。
接下来,设置了一些类变量和实例变量,包括 `root`、`dataNames`、`transform`、`loader`、`index`、`datalen`、`dataBuffer`、`fileIndx`、`TreePoint` 和 `fileLen`。
最后,通过断言确保找到了至少一个文件,否则抛出异常。
值得注意的是,在构造函数中还有一行被注释掉的代码:`self.dataLenPerFile = self.calcdataLenPerFile()`。它调用了一个名为 `calcdataLenPerFile()` 的方法来计算每个 'mat' 文件中的八叉树节点数量,并将结果赋给 `self.dataLenPerFile`。你可以在运行了 `calcdataLenPerFile()` 方法后,将其注释掉,然后直接使用给定的 `dataLenPerFile` 参数来指定值。
这段代码创建了一个自定义的数据集类,并提供了一些便捷的属性和方法来处理图像数据集。
阅读全文
相关推荐
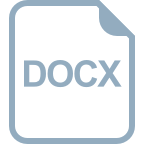
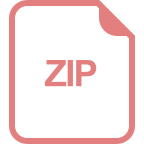
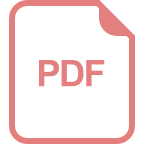

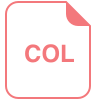











