Vue3中js实现在有限高度中显示列表:列表自动往上滚动显示,鼠标放上去后停止滚动,列表可鼠标滚轮显示剩下的内容,滚动时需要连续滚动,不要出现空白
时间: 2024-02-17 21:04:12 浏览: 19
可以使用Vue3的组件来实现在有限高度中显示列表,并且自动滚动和鼠标悬停停止滚动的效果。具体实现步骤如下:
1. 创建一个Vue3组件,定义一个data属性用来存储列表数据和滚动状态,以及一个scroll方法用来控制滚动。
```javascript
<template>
<div class="scroll-container" @mouseover="stopScroll" @mouseleave="startScroll" @wheel="scroll">
<div class="scroll-content" :style="{ transform: 'translateY(' + translateY + 'px)' }">
<div v-for="(item, index) in list" :key="index">
{{ item }}
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
list: [], // 列表数据
scrollHeight: 0, // 滚动区域高度
contentHeight: 0, // 滚动内容总高度
translateY: 0, // 滚动的Y轴距离
scrollTimer: null, // 滚动计时器
stopScrollFlag: false // 鼠标悬停停止滚动的标志
};
},
methods: {
// 添加列表数据
addItem(item) {
this.list.push(item);
this.contentHeight += 30; // 假设每个列表项高度为30px
},
// 开始滚动
startScroll() {
if (!this.scrollTimer) {
this.scrollTimer = setInterval(() => {
if (!this.stopScrollFlag) {
this.translateY -= 1;
if (this.translateY < this.scrollHeight - this.contentHeight) {
this.translateY = this.scrollHeight - this.contentHeight;
}
}
}, 10);
}
},
// 停止滚动
stopScroll() {
this.stopScrollFlag = true;
},
// 滚动列表
scroll(e) {
const delta = e.deltaY > 0 ? 1 : -1;
this.translateY += delta * 10;
if (this.translateY < this.scrollHeight - this.contentHeight) {
this.translateY = this.scrollHeight - this.contentHeight;
}
if (this.translateY > 0) {
this.translateY = 0;
}
e.preventDefault();
}
}
};
</script>
```
2. 在组件的mounted方法中,获取滚动区域的高度,以及列表项的总高度。
```javascript
mounted() {
const container = this.$el.querySelector('.scroll-container');
const content = this.$el.querySelector('.scroll-content');
this.scrollHeight = container.clientHeight;
this.contentHeight = content.clientHeight;
}
```
3. 在需要添加列表项的地方,调用组件的addItem方法,添加列表项。
```javascript
<template>
<div>
<button @click="addList">添加列表项</button>
<scroll-list ref="scrollList"></scroll-list>
</div>
</template>
<script>
import ScrollList from './ScrollList.vue';
export default {
components: {
ScrollList
},
methods: {
addList() {
const item = '列表项 ' + (this.$refs.scrollList.list.length + 1);
this.$refs.scrollList.addItem(item);
}
}
};
</script>
```
4. 在需要使用该组件的地方,引入组件并使用即可。
```javascript
<template>
<div>
<button @click="addList">添加列表项</button>
<scroll-list ref="scrollList"></scroll-list>
</div>
</template>
<script>
import ScrollList from './ScrollList.vue';
export default {
components: {
ScrollList
},
methods: {
addList() {
const item = '列表项 ' + (this.$refs.scrollList.list.length + 1);
this.$refs.scrollList.addItem(item);
}
}
};
</script>
```
通过以上步骤,就可以实现在有限高度中显示列表,并且自动滚动和鼠标悬停停止滚动的效果,同时也支持鼠标滚轮滚动显示剩下的内容,滚动时也不会出现空白。
相关推荐
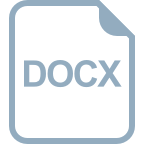
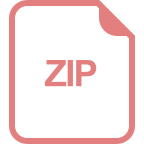














