在Django Rest Framework中,models.py中有一个TestPaper模型中有三个字段分别是:”question“用于连接Question模型的外键;”title“charfield的标题;”student“用于连接Student模型的外键。现在你需要在views.py中写入一个action,使用ModelViewSet中自带的创建功能进行对模型”POST“的接口。
时间: 2023-11-22 11:54:25 浏览: 38
好的,我可以帮你编写这个action。首先,在views.py中导入以下模块:
```python
from rest_framework import viewsets
from rest_framework.response import Response
from .models import TestPaper
from .serializers import TestPaperSerializer
```
接下来,定义一个TestPaperViewSet类继承自ModelViewSet,并设置queryset和serializer_class:
```python
class TestPaperViewSet(viewsets.ModelViewSet):
queryset = TestPaper.objects.all()
serializer_class = TestPaperSerializer
```
现在我们可以编写创建TestPaper模型实例的action了。在TestPaperViewSet类中添加以下方法:
```python
def create(self, request, *args, **kwargs):
serializer = self.get_serializer(data=request.data)
serializer.is_valid(raise_exception=True)
# 获取question和student对象
question_id = request.data.get('question')
student_id = request.data.get('student')
try:
question = Question.objects.get(id=question_id)
student = Student.objects.get(id=student_id)
except Question.DoesNotExist or Student.DoesNotExist:
return Response({'message': 'Invalid question or student ID'})
# 创建TestPaper实例并保存
test_paper = TestPaper(
question=question,
title=request.data.get('title'),
student=student
)
test_paper.save()
headers = self.get_success_headers(serializer.data)
return Response(serializer.data, status=201, headers=headers)
```
这个方法首先会使用serializer对请求数据进行验证,如果数据无效则返回400 Bad Request的响应。接着它会根据请求数据中的question和student字段获取对应的Question和Student对象,如果ID无效则返回404 Not Found的响应。最后,它会创建一个TestPaper实例并保存到数据库中,返回201 Created的响应。
注意,这个方法并没有覆盖ModelViewSet自带的create方法,所以在使用POST请求创建TestPaper实例时,仍然会调用ModelViewSet自带的create方法。只有当在TestPaperViewSet中定义了create方法时,才会调用TestPaperViewSet中的create方法。
相关推荐
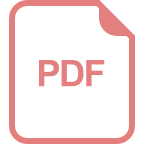
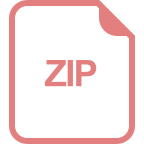
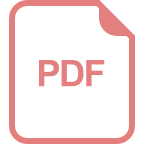














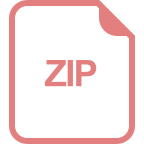