用Java编写一个程序将文件source.txt的内容复制到文件object.txt中,源文件和目标文件的名称在程序运行时输入
时间: 2024-12-31 20:47:17 浏览: 6
在Java中,你可以使用`java.io`包下的`FileInputStream`, `FileOutputStream`和`BufferedReader`/`BufferedWriter`类来读取源文件并将其内容写入目标文件。这里是一个简单的示例程序:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
try {
// 获取用户输入的源文件名
Scanner scanner = new Scanner(System.in);
System.out.println("请输入源文件(source.txt)的路径:");
String sourcePath = scanner.nextLine();
// 获取用户输入的目标文件名
System.out.println("请输入目标文件(object.txt)的路径:");
String outputPath = scanner.nextLine();
// 创建文件对象
FileInputStream fis = new FileInputStream(sourcePath);
FileOutputStream fos = new FileOutputStream(outputPath);
// 创建缓冲流
byte[] buffer = new byte[1024];
int length;
// 逐字节读取并写入目标文件
while ((length = fis.read(buffer)) != -1) {
fos.write(buffer, 0, length);
}
// 关闭所有流
fis.close();
fos.close();
System.out.println("源文件已成功复制到目标文件。");
} catch (FileNotFoundException e) {
e.printStackTrace();
System.out.println("文件未找到,请检查文件路径是否正确。");
} catch (IOException e) {
e.printStackTrace();
System.out.println("发生错误,无法复制文件,请检查是否有权限访问文件。");
}
}
}
```
在这个程序中,首先提示用户输入源文件和目标文件的路径,然后尝试打开它们并创建输入输出流。接着读取源文件的内容,并逐块写入到目标文件中。最后记得关闭所有流。
阅读全文
相关推荐








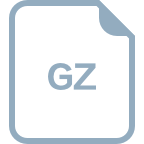

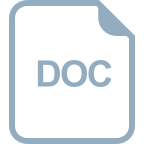







