栈 Stack 是一种先入后出的数据结构,最先入栈的元素称为栈底,最后入栈的元素称为栈顶。为了方便,可用 node.hpp 中的链表结构实现栈,并用链表头指向栈顶元素。 根据下述定义,请实现一个类模板 Stack ,使其可以保存不同类型的数据。 template <typename ElementType> //genericStack.h class Stack{ public: Stack(); ~Stack(); void push(ElementType obj); //将
时间: 2024-03-15 15:43:07 浏览: 52
元素 obj 压入栈中
ElementType pop(); //从栈中弹出并返回栈顶元素
bool isEmpty(); //判断栈是否为空
int size(); //返回栈的元素个数
private:
struct Node{
ElementType data;
Node* next;
Node(ElementType d, Node* n = NULL){
data = d;
next = n;
}
};
Node* top;
int count;
};
其中,push() 函数将元素 obj 压入栈中,pop() 函数从栈中弹出并返回栈顶元素,isEmpty() 函数用于判断栈是否为空,size() 函数返回栈的元素个数。
相关问题
描述 栈 Stack 是一种先入后出的数据结构,最先入栈的元素称为栈底,最后入栈的元素称为栈顶。为了方便,可用 node.hpp 中的链表结构实现栈,并用链表头指向栈顶元素。 根据下述定义,请实现一个类模板 Stack ,使其可以保存不同类型的数据。 template <typename ElementType> //genericStack.h class Stack{ public: Stack(); ~Stack(); void push(ElementType obj); //将新元素入栈 void pop(); //将栈顶元素出栈 ElementType getTop() const; //返回栈顶元素 bool isEmpty() const; //判断栈是否为空 private: struct Node{ // 栈结点类型 ElementType element; // 结点中存放的元素 Node *next; // 指向下一结点的指针 }; Node *top; // 栈顶 } template <typename ElementType> //genericStack.h class Stack{ public: Stack(); ~Stack(); void push(ElementType obj); //将新元素入栈 void pop(); //将栈顶元素出栈 ElementType getTop() const; //返回栈顶元素 bool isEmpty() const; //判断栈是否为空 private: struct Node{ // 栈结点类型 ElementType element; // 结点中存放的元素 Node *next; // 指向下一结点的指针 }; Node *top; // 栈顶 } Sample Output 8 7 6 5 4 3 2 1
以下是实现栈的代码示例:
```cpp
#include "node.hpp"
template <typename ElementType>
class Stack{
public:
Stack() {
top = nullptr; // 初始化栈顶指针为空
}
~Stack() {
while (!isEmpty()) {
pop(); // 释放所有结点
}
}
void push(ElementType obj) {
Node *newNode = new Node; // 创建一个新结点
newNode->element = obj; // 将元素存储到结点中
newNode->next = top; // 新结点的 next 指针指向当前的栈顶
top = newNode; // 更新栈顶指针
}
void pop() {
if (!isEmpty()) {
Node *temp = top; // 保存当前的栈顶指针
top = top->next; // 更新栈顶指针
delete temp; // 释放当前的栈顶结点
}
}
ElementType getTop() const {
if (!isEmpty()) {
return top->element; // 返回栈顶元素
}
throw std::out_of_range("Stack is empty"); // 抛出异常
}
bool isEmpty() const {
return top == nullptr; // 如果栈顶指针为空,则认为栈为空
}
private:
struct Node{
ElementType element;
Node *next;
};
Node *top;
};
```
使用示例:
```cpp
#include <iostream>
#include "genericStack.h"
int main() {
Stack<int> s;
for (int i = 1; i <= 8; i++) {
s.push(i); // 将 1 到 8 入栈
}
while (!s.isEmpty()) {
std::cout << s.getTop() << " "; // 输出栈顶元素
s.pop(); // 出栈
}
std::cout << std::endl; // 换行
return 0;
}
```
输出结果为:
```
8 7 6 5 4 3 2 1
```
#include "genericStack.hpp" #include <iostream> using namespace std; int main() //程序EX6_4.cpp { Stack<int> stack; // 实例化一个保存int型元素的栈 for (int i = 1; i < 9; i++) // 向栈中压入8个元素 stack.push(i); while (!stack.isEmpty()) { // 栈不为空时循环 cout << stack.getTop() << " "; // 显示栈顶元素 stack.pop(); // 弹出栈顶元素 } return 0; }栈 Stack 是一种先入后出的数据结构,最先入栈的元素称为栈底,最后入栈的元素称为栈顶。为了方便,可用 node.hpp 中的链表结构实现栈,并用链表头指向栈顶元素。 根据下述定义,请实现一个类模板 Stack ,使其可以保存不同类型的数据。 template <typename ElementType> //genericStack.h class Stack{ public: Stack(); ~Stack(); void push(ElementType obj); //将新元素入栈 void pop(); //将栈顶元素出栈 ElementType getTop() const; //返回栈顶元素 bool isEmpty() const; //判断栈是否为空 private: struct Node{ // 栈结点类型 ElementType element; // 结点中存放的元素 Node *next; // 指向下一结点的指针 }; Node *top; // 栈顶 } template <typename ElementType> //genericStack.h class Stack{ public: Stack(); ~Stack(); void push(ElementType obj); //将新元素入栈 void pop(); //将栈顶元素出栈 ElementType getTop() const; //返回栈顶元素 bool isEmpty() const; //判断栈是否为空 private: struct Node{ // 栈结点类型 ElementType element; // 结点中存放的元素 Node *next; // 指向下一结点的指针 }; Node *top; // 栈顶 }
以下是实现了上述定义的`Stack`类模板的代码:
```c++
#include <iostream>
using namespace std;
template <typename ElementType>
class Stack {
public:
Stack() {
top = nullptr;
}
~Stack() {
Node* temp;
while (top != nullptr) {
temp = top;
top = top->next;
delete temp;
}
}
void push(ElementType obj) {
Node* newNode = new Node();
newNode->element = obj;
newNode->next = top;
top = newNode;
}
void pop() {
if (isEmpty()) {
cout << "Stack is empty!" << endl;
return;
}
Node* temp = top;
top = top->next;
delete temp;
}
ElementType getTop() const {
if (isEmpty()) {
cout << "Stack is empty!" << endl;
return ElementType();
}
return top->element;
}
bool isEmpty() const {
return top == nullptr;
}
private:
struct Node {
ElementType element;
Node* next;
};
Node* top;
};
```
在这个代码中,我们定义了一个类模板`Stack`,其中`ElementType`是要保存在堆栈中的元素类型。我们使用一个内部结构体`Node`表示堆栈中的一个节点,其中`element`保存元素值,`next`指向下一个节点。`top`指向堆栈的顶部节点。
在`Stack`类模板中,我们实现了`push`、`pop`、`getTop`和`isEmpty`等公共函数。`push`函数将新元素入栈,`pop`函数将栈顶元素出栈,`getTop`函数返回栈顶元素,`isEmpty`函数判断栈是否为空。在`push`函数中,我们创建一个新的节点,将其指向当前的`top`节点,然后将`top`指针指向新的节点。在`pop`函数中,我们删除顶部节点,并将`top`指针指向下一个节点。在`getTop`函数中,我们返回顶部节点的元素值。在`isEmpty`函数中,我们检查`top`指针是否为空来判断堆栈是否为空。
在`main`函数中,我们实例化了一个保存`int`类型元素的堆栈对象`stack`,并向其中压入了8个元素。然后,我们使用`getTop`函数获取栈顶元素,并使用`pop`函数弹出栈顶元素,直到堆栈为空。
阅读全文
相关推荐
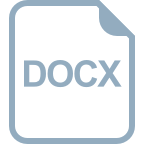
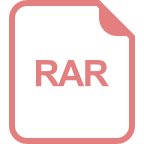
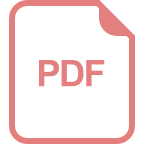
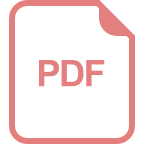
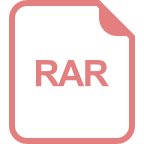
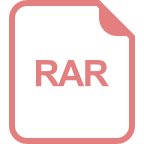
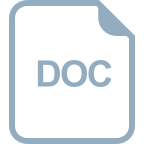
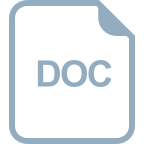
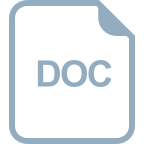
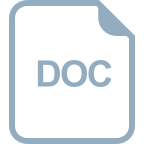
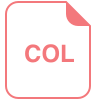


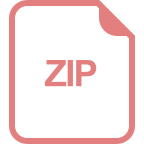