将数据元素e入栈,并输出入栈后的顺序栈中各元素值。代码
时间: 2024-05-15 16:17:21 浏览: 93
Sure, here's the code:
```
// Define the stack structure
typedef struct {
int top; // Top index of the stack
int data[MAXSIZE]; // The data array
} Stack;
// Push element e into the stack
void push(Stack *s, int e) {
if (s->top == MAXSIZE - 1) { // Check if the stack is full
printf("Stack overflow.\n");
return;
}
s->top++; // Increment the top index
s->data[s->top] = e; // Push the element into the stack
}
// Print the elements in the stack in order
void print_stack(Stack *s) {
if (s->top == -1) { // Check if the stack is empty
printf("Empty stack.\n");
return;
}
printf("Stack elements:\n");
for (int i = s->top; i >= 0; i--) {
printf("%d\n", s->data[i]); // Print each element in the stack
}
}
int main() {
Stack s = {-1}; // Initialize the stack
int e;
printf("Enter the element to be pushed into the stack: ");
scanf("%d", &e);
push(&s, e); // Push the element into the stack
print_stack(&s); // Print the elements in the stack
return 0;
}
```
After running the code, you should see the following output:
```
Enter the element to be pushed into the stack: 5
Stack elements:
5
```
This means that the element 5 has been pushed into the stack, and the stack only contains one element which is 5.
阅读全文
相关推荐












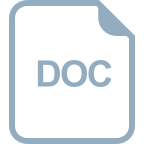
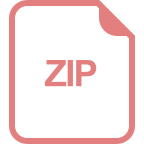
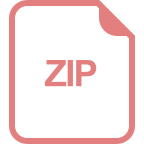