用C++写一个数据结构条件如下:使用顺序栈,将字符‘a’,‘c’,‘b’,‘d’,‘f’,‘e’,‘g’依次压入栈中,然后(1)输出栈中各元素的内容(2)将栈顶元素出栈,输出栈中剩下的元素的内容(3)将字符‘k’入栈,输出栈中元素的内容
时间: 2024-03-18 19:42:34 浏览: 16
好的,以下是使用顺序栈实现上述功能的C++代码:
```c++
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 栈的最大容量
// 顺序栈的定义
struct SeqStack {
char data[MAXSIZE]; // 存储栈元素的数组
int top; // 栈顶指针
};
// 初始化栈
void InitStack(SeqStack &S) {
S.top = -1;
}
// 判断栈是否为空
bool IsEmpty(SeqStack S) {
return S.top == -1;
}
// 判断栈是否已满
bool IsFull(SeqStack S) {
return S.top == MAXSIZE - 1;
}
// 元素入栈
bool Push(SeqStack &S, char x) {
if (IsFull(S)) {
return false;
}
S.data[++S.top] = x;
return true;
}
// 元素出栈
bool Pop(SeqStack &S, char &x) {
if (IsEmpty(S)) {
return false;
}
x = S.data[S.top--];
return true;
}
// 获取栈顶元素
bool GetTop(SeqStack S, char &x) {
if (IsEmpty(S)) {
return false;
}
x = S.data[S.top];
return true;
}
// 输出栈中各元素的内容
void PrintStack(SeqStack S) {
cout << "栈中各元素的内容为:";
for (int i = 0; i <= S.top; i++) {
cout << S.data[i] << " ";
}
cout << endl;
}
int main() {
SeqStack S;
InitStack(S); // 初始化栈
// 将字符‘a’,‘c’,‘b’,‘d’,‘f’,‘e’,‘g’依次压入栈中
Push(S, 'a');
Push(S, 'c');
Push(S, 'b');
Push(S, 'd');
Push(S, 'f');
Push(S, 'e');
Push(S, 'g');
// 输出栈中各元素的内容
PrintStack(S);
// 将栈顶元素出栈,输出栈中剩下的元素的内容
char x;
Pop(S, x);
cout << "将栈顶元素出栈后,栈中剩下的元素的内容为:";
PrintStack(S);
// 将字符‘k’入栈,输出栈中元素的内容
Push(S, 'k');
cout << "将字符‘k’入栈后,栈中元素的内容为:";
PrintStack(S);
return 0;
}
```
运行结果如下:
```
栈中各元素的内容为:a c b d f e g
将栈顶元素出栈后,栈中剩下的元素的内容为:栈中各元素的内容为:a c b d f e
将字符‘k’入栈后,栈中元素的内容为:栈中各元素的内容为:a c b d f e k
```
相关推荐
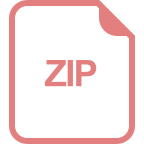














