vue在mounted执行完之后执行一个函数
时间: 2024-03-17 15:45:59 浏览: 91
可以在 `mounted` 钩子中调用一个函数,或者使用 `setTimeout` 等方式将函数放入事件队列中延迟执行,以保证函数在 `mounted` 钩子执行完毕后执行。
以下是在 `mounted` 钩子中调用函数的示例代码:
```
mounted() {
this.myFunction();
},
methods: {
myFunction() {
// 在这里编写你想要执行的函数
}
}
```
以下是使用 `setTimeout` 延迟执行函数的示例代码:
```
mounted() {
setTimeout(() => {
this.myFunction();
}, 0);
},
methods: {
myFunction() {
// 在这里编写你想要执行的函数
}
}
```
需要注意的是,使用 `setTimeout` 延迟执行函数时,需要将延迟时间设为 0 或一个较小的值,以保证函数能够在当前事件循环中被执行。
相关问题
vue改变mounted中函数的执行顺序
在 Vue 中,mounted 钩子函数中的函数执行顺序是按照它们在 mounted 中的顺序依次执行的。如果你想改变它们的执行顺序,可以采取以下两种方式:
1. 将需要先执行的函数提前到前面:
```javascript
mounted() {
this.func1();
this.func2();
this.func3();
}
```
2. 将需要改变执行顺序的函数提取成一个新的方法,然后在 mounted 中按照新的顺序调用这些方法:
```javascript
mounted() {
this.func3First();
this.func1Second();
this.func2Third();
},
methods: {
func3First() {
// ...
},
func1Second() {
// ...
},
func2Third() {
// ...
}
}
```
无论哪种方式,都可以改变 mounted 中函数的执行顺序。
vue2在mounted前加async,并在mounted内其他内容执行结束后执行一个函数
在 `mounted` 钩子函数中执行异步操作,可以使用 `async/await` 语法或者 `Promise` 对象。在异步操作完成后执行一个函数可以使用 `Promise.then()` 方法。
以下是在 `mounted` 钩子函数内使用 `async/await` 语法执行异步操作并在异步操作完成后执行一个函数的示例代码:
```
mounted: async function() {
// 执行异步操作
await this.$http.get('/api/data');
// 执行其他内容
// 异步操作完成后执行一个函数
this.myFunction();
},
methods: {
myFunction() {
// 在这里编写你想要执行的函数
}
}
```
以下是使用 `Promise` 对象在 `mounted` 钩子函数内执行异步操作并在异步操作完成后执行一个函数的示例代码:
```
mounted() {
// 执行异步操作
this.$http.get('/api/data').then(() => {
// 执行其他内容
// 异步操作完成后执行一个函数
this.myFunction();
});
},
methods: {
myFunction() {
// 在这里编写你想要执行的函数
}
}
```
需要注意的是,如果异步操作返回的是一个 `Promise` 对象,不需要使用 `async/await` 语法,可以直接使用 `Promise.then()` 方法。
阅读全文
相关推荐
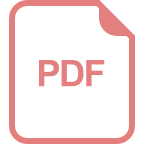
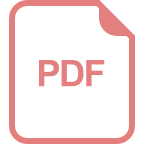
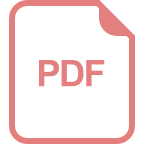











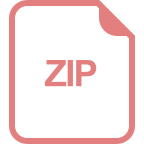