如何实现excel的导入导出请给出代码
时间: 2024-05-14 22:13:10 浏览: 36
以下是Java代码实现Excel的导入导出:
Excel导入:
```java
public List<List<String>> readExcel(String filePath) throws Exception {
List<List<String>> result = new ArrayList<>();
Workbook workbook = null;
try {
workbook = WorkbookFactory.create(new File(filePath));
Sheet sheet = workbook.getSheetAt(0);
for (int i = sheet.getFirstRowNum(); i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
if (row == null) {
continue;
}
List<String> rowList = new ArrayList<>();
for (int j = row.getFirstCellNum(); j <= row.getLastCellNum(); j++) {
Cell cell = row.getCell(j);
if (cell == null) {
continue;
}
rowList.add(cell.toString());
}
result.add(rowList);
}
} finally {
if (workbook != null) {
workbook.close();
}
}
return result;
}
```
Excel导出:
```java
public void writeExcel(String filePath, List<List<String>> data) throws Exception {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet();
for (int i = 0; i < data.size(); i++) {
Row row = sheet.createRow(i);
List<String> rowList = data.get(i);
for (int j = 0; j < rowList.size(); j++) {
Cell cell = row.createCell(j);
cell.setCellValue(rowList.get(j));
}
}
FileOutputStream outputStream = null;
try {
outputStream = new FileOutputStream(filePath);
workbook.write(outputStream);
} finally {
if (outputStream != null) {
outputStream.close();
}
if (workbook != null) {
workbook.close();
}
}
}
```
需要注意的是,需要引入Apache POI库才能使用以上代码。
阅读全文
相关推荐
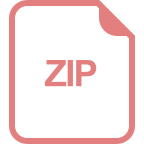
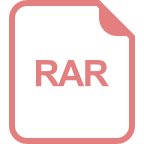
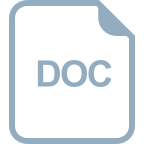
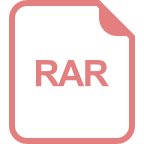
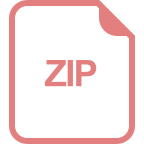
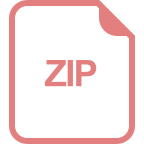
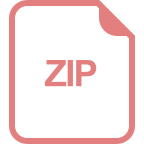
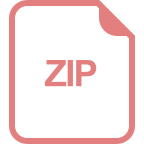
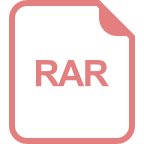
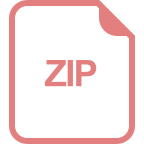
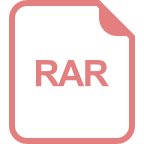
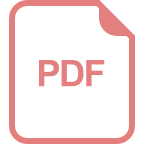
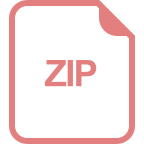
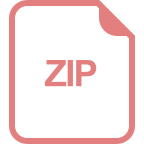
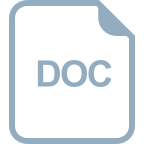
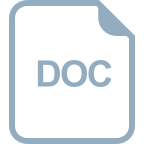