java正则替换字符串
时间: 2023-07-06 15:26:35 浏览: 111
可以使用Java中的String类的replaceAll()方法来替换字符串。该方法接受两个参数,第一个参数是一个正则表达式,第二个参数是替换后要放入的字符串。示例如下:
```java
String str = "Hello, world! This is a test string.";
String newStr = str.replaceAll("world", "Java");
System.out.println(newStr); // 输出:Hello, Java! This is a test string.
```
在上面的例子中,我们把字符串中的"world"替换成了"Java"。需要注意的是,replaceAll()方法返回的是一个新的字符串,原来的字符串并没有发生改变。
相关问题
Java用正则替换字符串中所有空格
你可以使用 Java 的 String 类中的 replaceAll() 方法来替换字符串中的所有空格。正则表达式中的空格表示为 "\\s"。下面是一个示例代码:
```java
String str = "Hello World! This is a string with spaces.";
String replacedStr = str.replaceAll("\\s", "");
System.out.println(replacedStr);
```
运行以上代码,输出将会是:
```
HelloWorld!Thisisastringwithspaces.
```
在这个示例中,我们使用 `str.replaceAll("\\s", "")` 将字符串 `str` 中的所有空格替换为空字符串,得到了替换后的字符串 `replacedStr`。
java正则表达式字符串匹配
Java正则表达式是一种强大的字符串匹配工具,可以用于在文本中查找符合特定模式的字符串。使用Java的正则表达式,你可以根据自己的需求定义匹配模式,并使用相应的方法来查找、替换或提取满足这一模式的字符串。
例如,可以使用Pattern类和Matcher类来进行正则表达式匹配。首先,使用Pattern.compile方法将正则表达式编译为一个Pattern对象,然后使用Matcher类的方法来执行匹配操作。
下面是一个示例代码,演示如何使用正则表达式匹配字符串:
String text = "Hello, world!";
String pattern = "wo\\w+"; // 匹配以wo开头的单词
Pattern regex = Pattern.compile(pattern);
Matcher matcher = regex.matcher(text);
while (matcher.find()) {
String match = matcher.group(); // 获取匹配到的字符串
System.out.println("匹配到的字符串:" + match);
}
以上代码将输出:wo
阅读全文
相关推荐
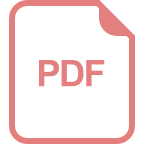
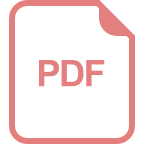
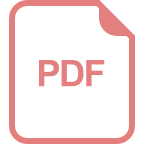
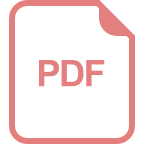
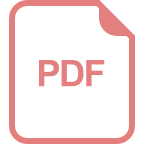
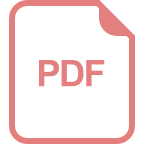
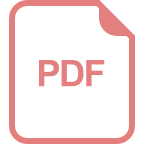
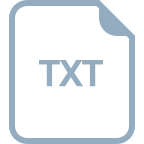
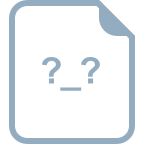
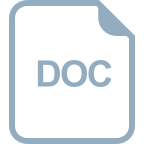





