正则替换指定字符串 java
时间: 2023-08-30 14:10:35 浏览: 92
可以使用Java的String类中的replace或replaceAll方法来实现正则替换指定字符串。比如:
```java
String originalString = "Hello, World! This is an example.";
String replacedString = originalString.replaceAll("example", "sample");
System.out.println(replacedString);
```
输出结果为:
```
Hello, World! This is an sample.
```
其中,replaceAll方法中第一个参数是一个正则表达式,第二个参数是要替换成的字符串。如果要替换多个匹配的字符串,可以使用正则表达式中的特殊符号,比如"|"表示或,或者在正则表达式中使用分组来匹配。
相关问题
正则替换指定格式字符串 java
答:你可以使用Java中的正则表达式来实现正则替换指定格式字符串。具体步骤为:首先在Java程序中定义一个正则表达式,然后使用Pattern和Matcher类来处理字符串,并使用replaceAll()方法来替换指定的字符串格式。例如,要将一个字符串中所有数字替换为”#”符号,可以使用以下代码:
```
String str = "1234 Hello 5678 World!";
Pattern pattern = Pattern.compile("\\d");
Matcher matcher = pattern.matcher(str);
String newStr = matcher.replaceAll("#");
System.out.println(newStr); //输出:"#### Hello #### World!"
```
其中,正则表达式“\d”表示匹配任意数字,替换成的字符串“#”表示将匹配到的数字替换为#符号。
正则表达式替换指定字符串
要使用正则表达式替换指定字符串,可以使用编程语言中的正则表达式相关函数。以下是一些示例:
Python:
```python
import re
text = "The quick brown fox jumped over the lazy dog"
new_text = re.sub(r"fox", "cat", text)
print(new_text)
```
输出:
```
The quick brown cat jumped over the lazy dog
```
Java:
```java
import java.util.regex.Matcher;
import java.util.regex.Pattern;
String text = "The quick brown fox jumped over the lazy dog";
Pattern pattern = Pattern.compile("fox", Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(text);
String new_text = matcher.replaceAll("cat");
System.out.println(new_text);
```
输出:
```
The quick brown cat jumped over the lazy dog
```
JavaScript:
```javascript
let text = "The quick brown fox jumped over the lazy dog";
let new_text = text.replace(/fox/gi, "cat");
console.log(new_text);
```
输出:
```
The quick brown cat jumped over the lazy dog
```
这些示例中,都使用了正则表达式来查找字符串中的指定文本,并将其替换为其他文本。具体的正则表达式语法和函数调用方式可能因编程语言而异。
阅读全文
相关推荐
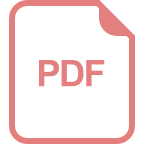
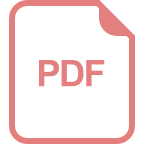
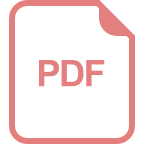
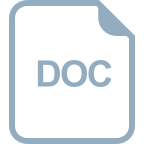






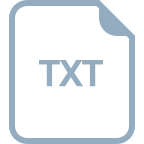
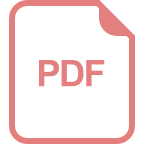
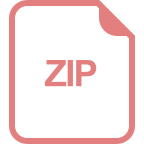
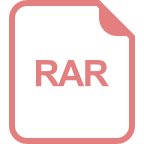
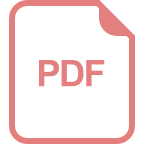
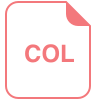