从客户端选择一张图片发送给服务器保存。 要求: 客户端:采用Qt5实现界面,先选择一张要发送的图片,然后点击“发送”按钮发送图片文件名和图片,通过thrift RPC方式通信 服务器:将接收到的图片保存。保存成功提示:保存图片成功,保存的文件名称为客户端发送的文件名称。通过thrift RPC方式通信.
时间: 2024-02-09 09:12:00 浏览: 134
以下是一个简单的实现,包括客户端和服务器端的代码。需要注意的是,这只是一个简单的示例,实际生产环境中需要考虑很多问题,比如错误处理、安全性、性能等等。
客户端代码:
```cpp
#include <QApplication>
#include <QtWidgets>
#include <thrift/transport/TSocket.h>
#include <thrift/transport/TBufferTransports.h>
#include <thrift/protocol/TBinaryProtocol.h>
#include "ImageTransfer.h"
using namespace apache::thrift;
using namespace apache::thrift::transport;
using namespace apache::thrift::protocol;
using namespace image_transfer;
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
// 创建文件选择对话框,选择要发送的图片文件
QFileDialog fileDialog;
fileDialog.setFileMode(QFileDialog::ExistingFile);
fileDialog.setNameFilter("Images (*.png *.xpm *.jpg *.bmp)");
if (fileDialog.exec() != QDialog::Accepted) {
return 0;
}
QString filePath = fileDialog.selectedFiles().first();
// 创建 RPC 客户端
boost::shared_ptr<TSocket> socket(new TSocket("localhost", 9090));
boost::shared_ptr<TTransport> transport(new TBufferedTransport(socket));
boost::shared_ptr<TProtocol> protocol(new TBinaryProtocol(transport));
ImageTransferClient client(protocol);
try {
// 连接 RPC 服务器
transport->open();
// 打开图片文件
QFile file(filePath);
if (!file.open(QIODevice::ReadOnly)) {
QMessageBox::critical(nullptr, "Error", "Failed to open file " + filePath);
return 1;
}
// 读取图片数据
QByteArray imageData = file.readAll();
// 发送图片文件名和数据到服务器
client.uploadImage(QFileInfo(filePath).fileName().toStdString(), imageData.constData(), imageData.size());
// 关闭连接
transport->close();
QMessageBox::information(nullptr, "Info", "Image sent successfully.");
} catch (TException& e) {
QMessageBox::critical(nullptr, "Error", "Failed to send image: " + QString::fromStdString(e.what()));
return 1;
}
return 0;
}
```
服务器端代码:
```cpp
#include <iostream>
#include <fstream>
#include <thrift/transport/TServerSocket.h>
#include <thrift/transport/TBufferTransports.h>
#include <thrift/protocol/TBinaryProtocol.h>
#include "ImageTransfer.h"
using namespace apache::thrift;
using namespace apache::thrift::transport;
using namespace apache::thrift::protocol;
using namespace image_transfer;
class ImageTransferHandler : public ImageTransferIf {
public:
void uploadImage(const std::string& fileName, const std::string& imageData, const int32_t size) {
std::ofstream file(fileName, std::ios::binary);
if (!file) {
std::cerr << "Failed to open file " << fileName << std::endl;
return;
}
file.write(imageData.c_str(), size);
file.close();
std::cout << "Image saved to file " << fileName << std::endl;
}
};
int main(int argc, char* argv[]) {
try {
// 创建 RPC 服务器
boost::shared_ptr<ImageTransferHandler> handler(new ImageTransferHandler());
boost::shared_ptr<TProcessor> processor(new ImageTransferProcessor(handler));
boost::shared_ptr<TServerTransport> serverTransport(new TServerSocket(9090));
boost::shared_ptr<TTransportFactory> transportFactory(new TBufferedTransportFactory());
boost::shared_ptr<TProtocolFactory> protocolFactory(new TBinaryProtocolFactory());
TSimpleServer server(processor, serverTransport, transportFactory, protocolFactory);
std::cout << "Server started" << std::endl;
// 启动服务器
server.serve();
} catch (TException& e) {
std::cerr << "Error: " << e.what() << std::endl;
return 1;
}
return 0;
}
```
需要注意的是,这里使用了 Thrift 的 TSimpleServer,它是一个简单的单线程服务器,适合测试和演示。在实际生产环境中,应该使用更强大的服务器,比如 TThreadedServer 或 TThreadPoolServer。
阅读全文
相关推荐









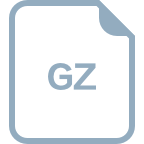








