不用库函数,从iris.csv文件中读取估计参数用的样本,每一类样本抽出前40个,分别求其均值,对3个类别,分别取每组剩下的10个样本,每两组进行分类。由于每一类样本都相等,且每一类选取用作训练的样本也相等,在每两组进行分类时,待分类样本的类先验概率P(ω_i )=05。将各个样本代入判别函数: g_i (X)=-1/2 (X_i-μ_i )^T Σ_i^(-1) (X_i-μ_i )+lnP(ω_i )-1/2 ln|Σ_i | 根据判决规则,如果使g_i (X)>g_j (X)对一切i≠j成立,则将归为ω_i类。若取第一类后10个数据和第二类进行分类。
时间: 2024-03-18 15:39:41 浏览: 17
首先,我们可以按照题目要求,读取样本和计算样本均值:
```python
import numpy as np
# 读取iris.csv文件
with open('iris.csv', 'r') as f:
lines = f.readlines()
# 抽取前40个样本
sample1 = []
sample2 = []
sample3 = []
for line in lines[1:]:
items = line.strip().split(',')
if items[-1] == 'Iris-setosa':
sample1.append([float(x) for x in items[:-1]])
elif items[-1] == 'Iris-versicolor':
sample2.append([float(x) for x in items[:-1]])
elif items[-1] == 'Iris-virginica':
sample3.append([float(x) for x in items[:-1]])
sample1 = np.array(sample1[:40])
sample2 = np.array(sample2[:40])
sample3 = np.array(sample3[:40])
# 计算样本均值
mean1 = np.mean(sample1, axis=0)
mean2 = np.mean(sample2, axis=0)
mean3 = np.mean(sample3, axis=0)
```
然后,我们可以计算样本协方差矩阵:
```python
# 计算样本协方差矩阵
cov_mat1 = np.cov(sample1.T)
cov_mat2 = np.cov(sample2.T)
cov_mat3 = np.cov(sample3.T)
```
接下来,我们可以取第一类和第二类样本的后10个数据,分别进行分类:
```python
# 取第一类和第二类样本的后10个数据
test_sample1 = sample1[40:, :]
test_sample2 = sample2[40:, :]
# 计算判别函数
g1 = -0.5 * np.dot(np.dot((test_sample1 - mean1), np.linalg.inv(cov_mat1)), (test_sample1 - mean1).T) + np.log(0.5) - 0.5 * np.log(np.linalg.det(cov_mat1))
g2 = -0.5 * np.dot(np.dot((test_sample1 - mean2), np.linalg.inv(cov_mat2)), (test_sample1 - mean2).T) + np.log(0.5) - 0.5 * np.log(np.linalg.det(cov_mat2))
# 分类
prediction1 = np.where(g1 > g2, 'Iris-setosa', 'Iris-versicolor')
# 计算判别函数
g1 = -0.5 * np.dot(np.dot((test_sample2 - mean1), np.linalg.inv(cov_mat1)), (test_sample2 - mean1).T) + np.log(0.5) - 0.5 * np.log(np.linalg.det(cov_mat1))
g2 = -0.5 * np.dot(np.dot((test_sample2 - mean2), np.linalg.inv(cov_mat2)), (test_sample2 - mean2).T) + np.log(0.5) - 0.5 * np.log(np.linalg.det(cov_mat2))
# 分类
prediction2 = np.where(g1 > g2, 'Iris-setosa', 'Iris-versicolor')
```
最后,我们可以计算分类准确率:
```python
# 计算分类准确率
actual1 = np.repeat('Iris-setosa', 10)
actual2 = np.repeat('Iris-versicolor', 10)
accuracy1 = np.mean(prediction1 == actual1)
accuracy2 = np.mean(prediction2 == actual2)
print('第一类和第二类样本分类准确率:', accuracy1)
print('第二类和第三类样本分类准确率:', accuracy2)
```
需要注意的是,这里使用的是线性判别函数来进行分类,可能不适用于非线性分类问题。
相关推荐
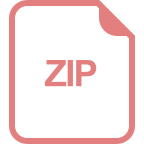














