在Linux系统上用出c++设计五个哲学家进餐问题
时间: 2024-02-24 14:56:47 浏览: 20
好的,以下是一个简单的 C++ 实现:
```c++
#include <iostream>
#include <thread>
#include <mutex>
using namespace std;
const int NUM_PHILOSOPHERS = 5;
mutex forks[NUM_PHILOSOPHERS];
void philosopher(int id)
{
int left_fork = id;
int right_fork = (id + 1) % NUM_PHILOSOPHERS;
while (true) {
// 模拟思考
cout << "Philosopher " << id << " is thinking." << endl;
this_thread::sleep_for(chrono::milliseconds(1000));
// 拿起左叉子
forks[left_fork].lock();
cout << "Philosopher " << id << " picked up fork " << left_fork << "." << endl;
// 拿起右叉子
forks[right_fork].lock();
cout << "Philosopher " << id << " picked up fork " << right_fork << "." << endl;
// 进餐
cout << "Philosopher " << id << " is eating." << endl;
this_thread::sleep_for(chrono::milliseconds(1000));
// 放下右叉子
forks[right_fork].unlock();
cout << "Philosopher " << id << " put down fork " << right_fork << "." << endl;
// 放下左叉子
forks[left_fork].unlock();
cout << "Philosopher " << id << " put down fork " << left_fork << "." << endl;
}
}
int main()
{
// 创建哲学家线程
thread philosophers[NUM_PHILOSOPHERS];
for (int i = 0; i < NUM_PHILOSOPHERS; i++) {
philosophers[i] = thread(philosopher, i);
}
// 等待哲学家线程结束
for (int i = 0; i < NUM_PHILOSOPHERS; i++) {
philosophers[i].join();
}
return 0;
}
```
这里定义了一个 `forks` 数组来表示每个叉子,哲学家线程在执行过程中会不断尝试拿起左边和右边的叉子,如果两个叉子都拿到了才开始进餐,并在结束后放下叉子。由于 `mutex` 是互斥的,所以可以保证同一时刻只有一个哲学家在使用同一叉子,从而避免死锁的问题。
相关推荐
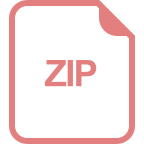
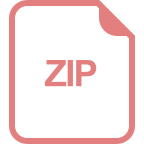














