用C++将24位bmp图像转换成8位unsigned char*不带bmp头的数据
时间: 2024-02-19 15:02:47 浏览: 298
可以按照以下步骤进行转换:
1. 打开24位bmp图像文件,并读取其像素数据。
2. 对于每个像素,将其RGB三个分量取平均值,得到一个灰度值。
3. 将每个灰度值按照8位无符号整数的格式存储到一个unsigned char*数组中。
4. 将该数组写入到一个新的文件中,不包括bmp头。
下面是一个实现的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
#pragma pack(push, 1)
struct BMPHeader {
uint16_t type;
uint32_t size;
uint16_t reserved1;
uint16_t reserved2;
uint32_t offset;
uint32_t header_size;
int32_t width;
int32_t height;
uint16_t planes;
uint16_t bit_count;
uint32_t compression;
uint32_t image_size;
int32_t x_pixels_per_meter;
int32_t y_pixels_per_meter;
uint32_t colors_used;
uint32_t important_colors;
};
#pragma pack(pop)
void write_data(const string& output_file, const unsigned char* data, size_t size) {
ofstream out(output_file, ios::out | ios::binary);
out.write((const char*)data, size);
out.close();
}
int main(int argc, char* argv[]) {
if (argc < 3) {
cout << "Usage: " << argv[0] << " input_file output_file" << endl;
return 1;
}
// 读取BMP文件头
BMPHeader header;
ifstream in(argv[1], ios::in | ios::binary);
in.read((char*)&header, sizeof(header));
// 读取像素数据
uint32_t data_size = header.width * header.height;
vector<unsigned char> pixel_data(data_size * 3);
in.read((char*)pixel_data.data(), pixel_data.size());
// 转换像素数据格式
vector<unsigned char> gray_data(data_size);
for (size_t i = 0; i < data_size; i++) {
unsigned char r = pixel_data[i * 3];
unsigned char g = pixel_data[i * 3 + 1];
unsigned char b = pixel_data[i * 3 + 2];
gray_data[i] = (r + g + b) / 3;
}
// 写入数据
write_data(argv[2], gray_data.data(), gray_data.size());
return 0;
}
```
注意,本示例代码只适用于没有调色板的24位BMP图像。如果图像中使用了调色板,需要根据调色板的颜色信息将每个像素转换为对应的颜色索引。
阅读全文
相关推荐
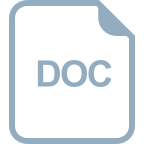
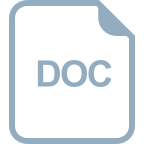
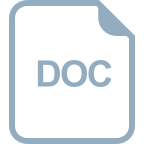
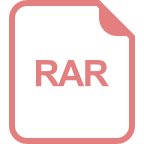
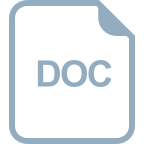
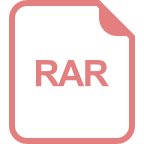
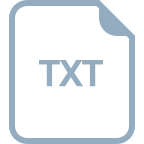
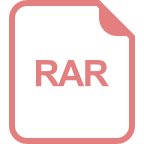
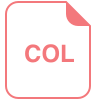









