python凯撒加密:将用户输入的一段文字(明文)变成密文输出。加密规则:明文中的每个字母替换为其后面第5个字母,其余字符不变。
时间: 2024-03-25 13:37:28 浏览: 181
好的,下面是Python实现凯撒加密的代码:
```python
def caesar_cipher(plain_text):
"""
将明文中的每个字母替换为其后面第5个字母,其余字符不变。
"""
cipher_text = ""
for char in plain_text:
if char.isalpha():
# 将字母转换为ASCII码,加上5,再转换回字母
cipher_char = chr((ord(char) - 97 + 5) % 26 + 97)
cipher_text += cipher_char
else:
cipher_text += char
return cipher_text
```
这个函数可以将输入的明文进行加密,返回加密后的密文。它首先遍历明文中的每个字符,如果是字母,则将其替换为后面第5个字母。这里使用了ASCII码进行转换,先将字母转换为ASCII码,加上5,再转换回字母即可。如果不是字母,则直接将其添加到密文中。最后返回加密后的密文。
相关问题
.凯撒加密:将用户输入的一段文字(明文)变成密文输出。加密规则:明文中的每个字母替换为其后面第5个字母,其余字符不变
好的,您的问题是凯撒加密的实现。我可以帮您写一个Python函数来实现凯撒加密,代码如下:
```python
def caesar_cipher(text):
cipher_text = ''
for letter in text:
if letter.isalpha():
shifted_letter = chr((ord(letter) - 97 + 5) % 26 + 97)
cipher_text += shifted_letter
else:
cipher_text += letter
return cipher_text
```
这个函数接受一个字符串参数,返回加密后的字符串。对于字符串中的每个字母,我们使用 ASCII 码将其转换为数字,再进行加密操作,最后将加密后的数字转换回字母。对于非字母字符,直接把它加入到密文中。
你是一个python大师,请你写出python代码要求如下:用户输入一段明文,程序输出相应的密文,并实现从密文恢复明文。 合格标准: 正确实现加密和解密算法,确保: 输入明文后,生成对应密文。 输入密文后,能正确还原出明文。
当然可以,我们可以使用简单的凯撒密码(Caesar Cipher)作为加密和解密算法示例,这是一种替换式密码,其中每个字母按照固定数量的位置向后移动。以下是Python代码实现:
```python
def caesar_cipher(text, shift=3):
encrypted_text = ""
for char in text:
if char.isalpha():
# 对于英文,只处理小写字母和大写字母
shift_amount = 65 if char.isupper() else 97
new_char_code = (ord(char) - shift_amount + shift) % 26 + shift_amount
encrypted_text += chr(new_char_code)
else:
encrypted_text += char
return encrypted_text
def encrypt(plaintext):
user_input = input("请输入明文: ")
return caesar_cipher(user_input)
def decrypt(ciphertext):
encrypted_user_input = input("请输入密文: ")
decrypted_text = caesar_cipher(encrypted_user_input, shift=-shift)
return decrypted_text
# 用户交互
print("加密过程:")
encrypted_text = encrypt("Hello, World!")
print("加密结果:", encrypted_text)
print("\n解密过程:")
decrypted_text = decrypt(encrypted_text)
print("解密结果:", decrypted_text)
```
这个代码会提示用户输入明文或密文,然后加密或解密。请注意,这是一个简单版本的凯撒密码,对于更复杂的加密需求,可能需要使用更专业的加密库,如`cryptography`模块。
阅读全文
相关推荐
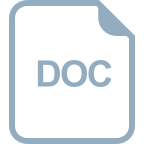
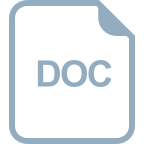
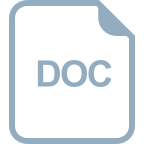













