编写程序实现分母为0和数组下标越界的异常及处理,使用常见的两种检测、捕捉处理的方法。java
时间: 2024-03-23 20:38:01 浏览: 90
在Java中,分母为0和数组下标越界都可以通过异常处理来解决。下面是两种处理方法:
方法一:使用try-catch块
```java
public class ExceptionHandlingExample {
public static void main(String[] args) {
int a = 5;
int b = 0;
int[] arr = {1, 2, 3};
try {
// 分母为0异常
int c = a / b;
// 数组下标越界异常
int d = arr[3];
} catch (ArithmeticException e) {
System.out.println("分母为0异常:" + e.getMessage());
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("数组下标越界异常:" + e.getMessage());
}
}
}
```
方法二:使用throws关键字
```java
public class ExceptionHandlingExample {
public static void main(String[] args) throws ArithmeticException, ArrayIndexOutOfBoundsException {
int a = 5;
int b = 0;
int[] arr = {1, 2, 3};
// 分母为0异常
int c = a / b;
// 数组下标越界异常
int d = arr[3];
}
}
```
在这两种方法中,当程序执行到出现异常的代码时,会抛出异常。在第一种方法中,我们通过try-catch块捕获异常并处理。在第二种方法中,我们使用throws关键字声明方法可能会抛出的异常,然后在调用该方法时使用try-catch块捕获异常。
阅读全文
相关推荐



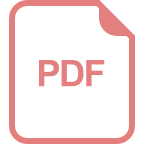









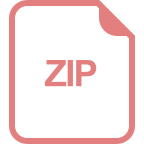

