解释代码 img_input = cv2.cvtColor(img_input,cv2.COLOR_GRAY2BGR)
时间: 2023-06-11 18:05:34 浏览: 94
这行代码将灰度图像转换为BGR格式的彩色图像。cv2.cvtColor是OpenCV中的一个函数,用于将图像从一种颜色空间转换为另一种颜色空间。在这里,图像被转换为BGR格式。色彩空间转换可以用于许多不同的图像处理应用,包括图像增强、目标检测和机器学习等。
相关问题
import numpy as np import cv2 Load image img = cv2.imread("input.jpg") Convert to grayscale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) Detect edges edges = cv2.Canny(gray, 100, 200) Display image with edges cv2.imshow("Image with Edges", edges) Automatically select edge points using HoughLines method lines = cv2.HoughLinesP(edges, 1, np.pi/180, threshold=100, minLineLength=50, maxLineGap=10) points = [] for line in lines: x1, y1, x2, y2 = line[0] points.append((x1, y1)) points.append((x2, y2)) Generate TSP art and tsplib dataset n = len(points) distances = np.zeros((n, n)) for i in range(n): for j in range(n): if i != j: distances[i][j] = np.sqrt((points[i][0] - points[j][0]) ** 2 + (points[i][1] - points[j][1]) ** 2) Write tsplib dataset with open("output.tsp", "w") as f: f.write("NAME: output\n") f.write("TYPE: TSP\n") f.write("DIMENSION: {}\n".format(n)) f.write("EDGE_WEIGHT_TYPE: EUC_2D\n") f.write("NODE_COORD_SECTION\n") for i in range(n): f.write("{} {} {}\n".format(i+1, points[i][0], points[i][1])) f.write("EOF\n") Display TSP art tsp_art = np.zeros_like(gray) path = list(range(n)) + [0] for i in range(n): cv2.line(tsp_art, points[path[i]], points[path[i+1]], (255, 255, 255), thickness=1) cv2.imshow("TSP Art", tsp_art) cv2.waitKey(0) cv2.destroyAllWindows()
这是一段使用OpenCV和NumPy库实现的图像处理代码,其目的是将一张图像转换成TSP艺术,并生成一个tsplib数据集。具体步骤如下:
1. 使用OpenCV的imread函数读取一张图像,将其转换为灰度图像。
2. 使用Canny函数检测边缘。
3. 使用HoughLinesP函数自动选择边缘点。
4. 使用NumPy计算两点之间的距离,生成距离矩阵。
5. 将距离矩阵写入tsplib数据集文件。
6. 使用OpenCV绘制TSP艺术,并显示。
值得注意的是,这段代码使用的是HoughLinesP函数自动选择边缘点,因此不一定能够得到最优的TSP艺术结果。如果需要更精确的结果,可以考虑手动选择边缘点,并使用更高效的TSP求解算法。
def deal_threshold(image): gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) Imgaepil = Image.fromarray(gray) # 边缘检测 edges = cv2.Canny(gray, 50, 150) # 使用霍夫变换检测直线 lines = cv2.HoughLines(edges, 1, np.pi / 180, threshold=70) sumang = 0 thresh = None if (lines is not None): for line in lines: anglep = line[0][1] * 180 / np.pi if (anglep > 90): anglep = 180 - anglep print(anglep) sumang = sumang + anglep angle = sumang / len(lines) thresh = Imgaepil.rotate(angle) return thresh def process_images(input_folder, output_folder): # 创建输出文件夹 os.makedirs(output_folder, exist_ok=True) # 遍历输入文件夹中的图片 for filename in os.listdir(input_folder): if filename.endswith(".jpg") or filename.endswith(".png") or filename.endswith(".JPG"): # 读取图片 image_path = os.path.join(input_folder, filename) image = cv2.imread(image_path) # 处理图片 thresh = deal_threshold(image) # 保存处理后的图片 output_path = os.path.join(output_folder, filename) cv2.imwrite(output_path, thresh) print(f"Processed {filename}") # 指定输入文件夹和输出文件夹 input_folder = "img_out_binary" output_folder = "rotated_img" # 调用函数进行处理 process_images(input_folder, output_folder),程序执行后提示 cv2.imwrite(output_path, thresh) cv2.error: OpenCV(4.7.0) D:\a\opencv-python\opencv-python\opencv\modules\imgcodecs\src\loadsave.cpp:783: error: (-215:Assertion failed) !_img.empty() in function 'cv::imwrite',优化程序
在你的代码中,出现了一个错误,即 `cv2.imwrite` 报错:`cv2.error: OpenCV(4.7.0) D:\a\opencv-python\opencv-python\opencv\modules\imgcodecs\src\loadsave.cpp:783: error: (-215:Assertion failed) !_img.empty() in function 'cv::imwrite'`。这个错误通常表示尝试保存一个空图像。
要优化你的程序,可以添加一些错误处理和调试步骤来排查问题。以下是一些可能的优化措施:
1. 检查 `deal_threshold` 函数中是否正确处理了所有情况。确保 `thresh` 不会为空,并且在返回之前对其进行了正确的处理。
2. 在 `process_images` 函数中,检查是否成功读取了图像并确保图像不为空。你可以尝试在读取图像之后添加一些条件语句,以确保图像加载成功。
3. 确保输出文件夹正确创建,并检查保存图像的路径是否正确。你可以添加一些调试语句来输出路径和变量的值,以确保它们符合预期。
4. 如果问题仍然存在,你可以尝试使用其他方法保存图像,例如使用PIL库(`Pillow`)中的 `Image.fromarray` 和 `Image.save` 函数代替 `cv2.imwrite`。
通过以上优化步骤,你应该能够解决保存图像时出现的错误。请记得在处理图像之前先检查图像的加载情况,并确保所有的变量和路径都正确。
阅读全文
相关推荐
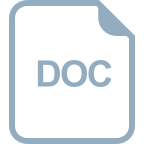
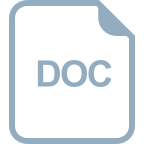
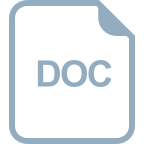













