输入10个学生5门课的成绩,分别用函数实现下列功能: ①计算每个学生的平均分; ②计算每门课的平均分; ③找出所有50个分数中最高的分数所对应的学生和课程; ④计算平均分方差: 其中,x:为某一学生的平均分。使用c++
时间: 2024-01-01 16:04:44 浏览: 91
以下是实现这些功能的C++代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
const int N = 10;
const int M = 5;
// 计算每个学生的平均分
void avgStuScore(float score[N][M], float avgStu[N]) {
for (int i = 0; i < N; i++) {
float sum = 0;
for (int j = 0; j < M; j++) {
sum += score[i][j];
}
avgStu[i] = sum / M;
}
}
// 计算每门课的平均分
void avgCourseScore(float score[N][M], float avgCourse[M]) {
for (int i = 0; i < M; i++) {
float sum = 0;
for (int j = 0; j < N; j++) {
sum += score[j][i];
}
avgCourse[i] = sum / N;
}
}
// 找出所有50个分数中最高的分数所对应的学生和课程
void findMaxScore(float score[N][M], int &stu, int &course) {
float maxScore = score[0][0];
for (int i = 0; i < N; i++) {
for (int j = 0; j < M; j++) {
if (score[i][j] > maxScore) {
maxScore = score[i][j];
stu = i;
course = j;
}
}
}
}
// 计算平均分方差
float avgScoreVariance(float score[N][M], float avg) {
float sum = 0;
for (int i = 0; i < N; i++) {
for (int j = 0; j < M; j++) {
sum += pow(score[i][j] - avg, 2);
}
}
return sum / (N * M);
}
int main() {
float score[N][M];
float avgStu[N];
float avgCourse[M];
int stu, course;
cout << "请输入每个学生的5门课成绩:" << endl;
for (int i = 0; i < N; i++) {
for (int j = 0; j < M; j++) {
cin >> score[i][j];
}
}
// 计算每个学生的平均分
avgStuScore(score, avgStu);
cout << "每个学生的平均分为:" << endl;
for (int i = 0; i < N; i++) {
cout << "第" << i+1 << "个学生的平均分为:" << avgStu[i] << endl;
}
// 计算每门课的平均分
avgCourseScore(score, avgCourse);
cout << "每门课的平均分为:" << endl;
for (int i = 0; i < M; i++) {
cout << "第" << i+1 << "门课的平均分为:" << avgCourse[i] << endl;
}
// 找出所有50个分数中最高的分数所对应的学生和课程
findMaxScore(score, stu, course);
cout << "最高分数为:" << score[stu][course] << endl;
cout << "最高分数所对应的学生为第" << stu+1 << "个学生" << endl;
cout << "最高分数所对应的课程为第" << course+1 << "门课" << endl;
// 计算平均分方差
float avgAll = 0;
for (int i = 0; i < N; i++) {
avgAll += avgStu[i];
}
avgAll /= N;
float variance = avgScoreVariance(score, avgAll);
cout << "平均分方差为:" << variance << endl;
return 0;
}
```
这里实现了四个函数,分别用来计算每个学生的平均分、每门课的平均分、找出所有50个分数中最高的分数所对应的学生和课程、计算平均分方差。在主函数中,先输入每个学生的5门课成绩,然后分别调用这四个函数,并输出结果。
阅读全文
相关推荐
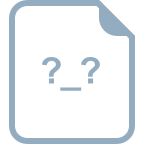















