c语言编程实现:输入 10 个学生 5 门课的成绩,分别用函数实现下列功能: 功能 1:计算每个学生的平均分; 功能 2:计算每门课的平均分; 功能 3:找出所有 50 个分数中最高的分数对应的学生和课程。
时间: 2024-01-01 22:05:52 浏览: 64
以下是C语言实现输入10个学生5门课程成绩的功能:
```c
#include <stdio.h>
// 计算每个学生的平均分
void calculateStudentAverage(float scores[][5], float studentAverage[], int numStudents, int numCourses) {
for (int i = 0; i < numStudents; i++) {
float sum = 0;
for (int j = 0; j < numCourses; j++) {
sum += scores[i][j];
}
studentAverage[i] = sum / numCourses; }
}
// 计算每门课的平均分
void calculateCourseAverage(float scores[][5], float courseAverage[], int numStudents, int numCourses) {
for (int j = 0; j < numCourses; j++) {
float sum = 0;
for (int i = 0; i < numStudents; i++) {
sum += scores[i][j];
}
courseAverage[j] = sum / numStudents;
}
}
// 找出最高分数所对应的学生和课程
void findHighestScore(float scores[][5], int numStudents, int numCourses, int *studentIndex, int *courseIndex) {
float highestScore = scores[0][0];
*studentIndex = 0;
*courseIndex = 0;
for (int i = 0; i < numStudents; i++) {
for (int j = 0; j < numCourses; j++) {
if (scores[i][j] > highestScore) {
highestScore = scores[i][j];
*studentIndex = i;
*courseIndex = j;
}
}
}
}
int main() {
float scores[10][5];
float studentAverage[10];
float courseAverage[5];
int highestStudentIndex, highestCourseIndex;
// 输入学生的成绩
printf("请输入10个学生5门课程的成绩:\n");
for (int i = 0; i < 10; i++) {
printf("请输入第%d个学生的成绩:\n", i+1);
for (int j = 0; j < 5; j++) {
scanf("%f", &scores[i][j]);
}
}
// 计算每个学生的平均分
calculateStudentAverage(scores, studentAverage, 10, 5);
// 计算每门课的平均分
calculateCourseAverage(scores, courseAverage, 10, 5);
// 找出最高分数所对应的学生和课程
findHighestScore(scores, 10, 5, &highestStudentIndex, &highestCourseIndex);
// 输出结果
printf("每个学生的平均分:\n");
for (int i = 0; i < 10; i++) {
printf("学生%d的平均分为:%.2f\n", i+1, studentAverage[i]);
}
printf("每门课的平均分:\n");
for (int j = 0; j < 5; j++) {
printf("课程%d的平均分为:%.2f\n", j+1, courseAverage[j]);
}
printf("最高分数所对应的学生和课程:\n");
printf("学生%d的课程%d得到了最高分数:%.2f\n", highestStudentIndex+1, highestCourseIndex+1, scores[highestStudentIndex][highestCourseIndex]);
return 0;
}
```
相关推荐
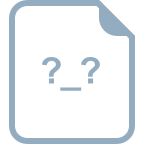
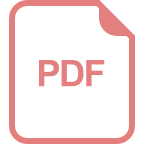
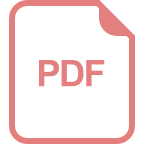













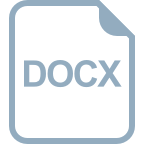
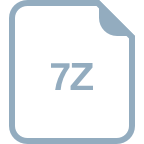