C语言编程实现 a) 调用pipe()创建无名管道 b) 调用fork创建一个子进程 c) 子进程 -用signal( )安装信号SIGALRM 在SIGALRM的信号处理函数内执行下列操作: 打印出pid 和接收到的信号值 向管道内写入所有的小写字母,打印出进程号及成功写入的字节数 -用signal( )安装信号SIGINT 在SIGINT的信号处理函数内执行下列操作: 打印出pid 和接收到的信号值 重新安装信号SIGINT,采用默认方式处理该信号 -设置一个定时器,2s后产生SIGALRM信号 -进入while(1)循环,每隔1s打印出一行” …child is waiting" d)父进程 -打印出进程号 -从管道读出数据,每次读8个字符,直到读出管道中的所有内容打印出每次读出的字节数及读出的内容 -向子进程发送信号SIGINT -等待1s -再次向子进程发送信号SIGINT -等待子进程结束 -父进程退出
时间: 2024-03-02 09:53:10 浏览: 112
好的,您想问的是如何使用 C 语言编写一个程序,实现创建无名管道、fork 子进程、设置信号处理函数、定时器、进程间通信、发送信号等功能,具体步骤如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/wait.h>
#include <signal.h>
#include <ctype.h>
#define BUF_SIZE 1024
void sig_alrm_handler(int signo);
void sig_int_handler(int signo);
int main() {
pid_t pid;
int pipefd[2];
char buf[BUF_SIZE];
int n, count = 0;
if (pipe(pipefd) < 0) {
perror("pipe error");
exit(1);
}
if ((pid = fork()) < 0) {
perror("fork error");
exit(1);
} else if (pid == 0) { // 子进程
close(pipefd[0]); // 关闭读端
// 安装 SIGALRM 信号处理函数
if (signal(SIGALRM, sig_alrm_handler) == SIG_ERR) {
perror("signal error");
exit(1);
}
// 设置定时器
alarm(2);
while (1) {
printf("... child is waiting\n");
sleep(1);
}
} else { // 父进程
close(pipefd[1]); // 关闭写端
// 从管道中读取数据
while ((n = read(pipefd[0], buf, BUF_SIZE)) > 0) {
for (int i = 0; i < n; i++) {
printf("%c", buf[i]);
if (islower(buf[i])) {
count++;
}
}
}
printf("\n%d lowercase letters are read from child\n", count);
// 发送 SIGINT 信号给子进程
if (kill(pid, SIGINT) < 0) {
perror("kill error");
exit(1);
}
// 等待 1 秒
sleep(1);
// 再次发送 SIGINT 信号给子进程
if (kill(pid, SIGINT) < 0) {
perror("kill error");
exit(1);
}
// 等待子进程结束
if (waitpid(pid, NULL, 0) < 0) {
perror("waitpid error");
exit(1);
}
}
return 0;
}
void sig_alrm_handler(int signo) {
printf("pid %d received signal %d\n", getpid(), signo);
char buf[26];
for (int i = 0; i < 26; i++) {
buf[i] = 'a' + i;
}
// 写入数据到管道
int n = write(pipefd[1], buf, 26);
printf("pid %d wrote %d bytes to pipe\n", getpid(), n);
// 重新设置定时器
alarm(2);
}
void sig_int_handler(int signo) {
printf("pid %d received signal %d\n", getpid(), signo);
// 恢复 SIGINT 的默认处理方式
if (signal(SIGINT, SIG_DFL) == SIG_ERR) {
perror("signal error");
exit(1);
}
}
```
这个程序的主要思路是:创建无名管道,fork 子进程,子进程设置定时器和 SIGALRM 信号处理函数,父进程从管道中读取数据,并向子进程发送 SIGINT 信号。具体实现细节请参考代码注释。
阅读全文
相关推荐

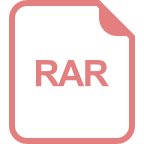
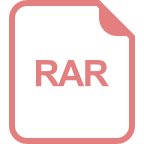




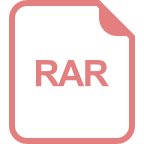
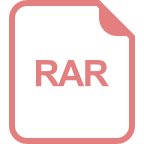








