编写一段程序,使用系统调用fork()创建两个子进程
时间: 2023-05-31 10:17:48 浏览: 653
### 回答1:
下面是一个使用系统调用fork()创建两个子进程的程序:
```
#include <stdio.h>
#include <unistd.h>
int main() {
pid_t pid1, pid2;
pid1 = fork();
if (pid1 == 0) {
// 子进程1
printf("I am child process 1, my pid is %d\n", getpid());
} else if (pid1 > 0) {
// 父进程
pid2 = fork();
if (pid2 == 0) {
// 子进程2
printf("I am child process 2, my pid is %d\n", getpid());
} else if (pid2 > 0) {
// 父进程
printf("I am parent process, my pid is %d\n", getpid());
} else {
// fork()失败
printf("fork() failed\n");
return 1;
}
} else {
// fork()失败
printf("fork() failed\n");
return 1;
}
return 0;
}
```
这个程序使用了两次fork()系统调用,首先创建了一个子进程1,然后在父进程中再创建一个子进程2。在每个子进程中,打印出自己的进程ID。在父进程中,也打印出自己的进程ID。
### 回答2:
fork()是Linux系统中的一个系统调用,它用于在当前进程中创建一个新的子进程。新的子进程是当前进程的完全复制,包含当前进程的所有信息和数据,因此它与当前进程共享程序代码、打开的文件、变量等。但是由于它是一个新的进程,因此它拥有自己的进程ID和资源使用情况。
编写一个程序使用fork()创建两个子进程,可以按照以下步骤进行:
1. 导入必要的头文件,包括unistd.h和stdio.h。其中unistd.h定义了fork()系统调用。
2. 在主函数中声明一个整型变量pid用来存储fork()的返回值,以便判断当前代码是运行在父进程中还是子进程中。
3. 调用fork()系统调用,创建两个子进程。每个子进程都有一个唯一的PID,可以用pid变量来区分父进程和子进程。
4. 在子进程的分支中,打印一条语句“这是子进程”,并使用exit()函数来结束该进程,因为子进程不需要继续执行。
5. 在父进程的分支中,打印一条语句“这是父进程”,并使用wait()函数等待子进程结束。wait()函数将使父进程阻塞,直到其中一个子进程结束。在子进程结束后,wait()函数将返回子进程的PID。
下面是完整的程序示例:
#include <unistd.h>
#include <stdio.h>
int main()
{
pid_t pid1, pid2;
pid1 = fork(); // 创建第一个子进程
if (pid1 == 0) // 子进程分支
{
printf("这是子进程1,进程ID=%d\n", getpid());
exit(0);
}
else // 父进程分支
{
printf("这是父进程,进程ID=%d\n", getpid());
pid2 = fork(); // 创建第二个子进程
if (pid2 == 0) // 子进程分支
{
printf("这是子进程2,进程ID=%d\n", getpid());
exit(0);
}
else // 父进程分支
{
printf("这是父进程,进程ID=%d\n", getpid());
wait(NULL); // 等待第一个子进程结束
wait(NULL); // 等待第二个子进程结束
}
}
return 0;
}
该程序会输出以下结果:
这是父进程,进程ID=1234
这是子进程1,进程ID=1235
这是父进程,进程ID=1234
这是子进程2,进程ID=1236
可以看到,父进程创建了两个子进程,每个子进程都输出了一行提示语句,并在结束后退出。父进程在创建完子进程后等待它们的结束,然后输出自己的提示语句,最终结束。
### 回答3:
编写一个程序,使用系统调用fork()创建两个子进程,每个子进程都打印自己的进程ID和父进程ID,然后使用exec()系统调用来运行另外一个程序。
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
int main() {
pid_t pid1, pid2;
int ret;
pid1 = fork(); // 创建第一个子进程
if (pid1 < 0) { // fork()失败
perror("fork");
exit(EXIT_FAILURE);
}
else if (pid1 == 0) { // 子进程1
printf("child 1 process ID: %d\n", getpid());
printf("child 1 parent process ID: %d\n", getppid());
ret = execl("/usr/bin/ls", "ls", "-l", NULL);
if (ret == -1) { // execl()运行失败
perror("execl");
exit(EXIT_FAILURE);
}
}
else { // 父进程
pid2 = fork(); // 创建第二个子进程
if (pid2 < 0) { // fork()失败
perror("fork");
exit(EXIT_FAILURE);
}
else if (pid2 == 0) { // 子进程2
printf("child 2 process ID: %d\n", getpid());
printf("child 2 parent process ID: %d\n", getppid());
ret = execl("/usr/bin/ps", "ps", "aux", NULL);
if (ret == -1) { // execl()运行失败
perror("execl");
exit(EXIT_FAILURE);
}
}
else { // 父进程
wait(NULL); // 等待子进程1结束
wait(NULL); // 等待子进程2结束
printf("parent process ID: %d\n", getpid());
printf("parent parent process ID: %d\n", getppid());
printf("both child processes have completed\n");
}
}
return 0;
}
程序首先调用fork()来创建第一个子进程,如果该调用失败,则打印错误消息并退出程序。如果调用成功,则子进程1打印自己的进程ID和父进程ID,然后调用execl()运行另一个程序(/usr/bin/ls)。如果execl()调用失败,则打印错误消息并退出子进程。
在父进程中,程序再次调用fork()来创建第二个子进程。如果调用失败,则打印错误消息并退出。如果调用成功,则子进程2打印自己的进程ID和父进程ID,然后调用execl()运行另一个程序(/usr/bin/ps)。如果execl()调用失败,则打印错误消息并退出子进程。
在父进程中,程序使用wait()函数等待两个子进程完成。一旦两个子进程都完成,父进程就打印自己的进程ID和父进程ID,并打印消息表明两个子进程都已经完成。程序随后退出。
相关推荐
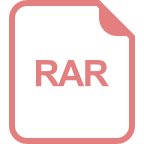
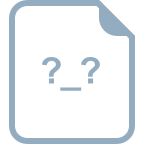
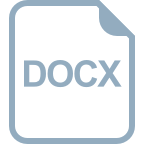














