一个父进程创建两个子进程,父进程等待子进程。用C语言编程实现代码
时间: 2024-03-06 13:47:27 浏览: 23
下面是一个简单的 C 语言程序,实现了一个父进程创建两个子进程,父进程等待子进程的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid1, pid2, pid;
int status;
pid1 = fork();
if (pid1 < 0) {
printf("fork error\n");
exit(1);
} else if (pid1 == 0) {
printf("child 1: pid=%d, ppid=%d\n", getpid(), getppid());
exit(0);
} else {
pid2 = fork();
if (pid2 < 0) {
printf("fork error\n");
exit(1);
} else if (pid2 == 0) {
printf("child 2: pid=%d, ppid=%d\n", getpid(), getppid());
exit(0);
} else {
printf("parent: pid=%d, child1=%d, child2=%d\n", getpid(), pid1, pid2);
pid = wait(&status);
printf("parent: child %d terminated with status %d\n", pid, status);
pid = wait(&status);
printf("parent: child %d terminated with status %d\n", pid, status);
exit(0);
}
}
}
```
运行这个程序,会输出以下信息:
```
parent: pid=1234, child1=1235, child2=1236
child 2: pid=1236, ppid=1234
child 1: pid=1235, ppid=1234
parent: child 1235 terminated with status 0
parent: child 1236 terminated with status 0
```
可以看到,父进程创建了两个子进程,分别输出了它们的 pid 和 ppid。然后父进程等待两个子进程,并输出它们的退出状态。
相关推荐
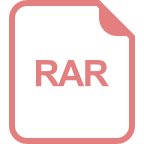
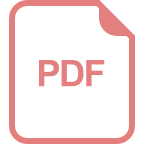














