html纵向渐变
时间: 2023-07-01 20:07:11 浏览: 54
你可以使用 CSS 的 linear-gradient() 函数来设置纵向渐变背景。例如,下面的代码会创建一个从上到下的红色到蓝色的渐变:
```html
<div style="background: linear-gradient(to bottom, red, blue); height: 200px;"></div>
```
其中,linear-gradient() 函数的第一个参数指定了渐变方向,to bottom 表示从上到下。接下来的参数是渐变的起点和终点颜色,可以根据自己的需求进行设置。在上面的例子中,起点为红色,终点为蓝色。最后,设置了 div 元素的高度为 200px,以便看到渐变效果。
你可以调整 linear-gradient() 函数的参数来实现不同的纵向渐变效果。例如,你可以改变方向、添加更多的颜色、调整颜色的位置等等。
相关问题
vue实现渐变色(纵向)
Vue可以使用CSS来实现渐变色(纵向)。你可以使用`v-bind:style`指令将CSS样式动态绑定到元素上。
首先,在Vue组件中,你可以定义一个data属性来存储渐变色的起始颜色和结束颜色,例如:
```javascript
data() {
return {
gradientStartColor: 'red',
gradientEndColor: 'blue'
}
}
```
然后,在模板中,你可以使用`v-bind:style`指令来绑定动态的CSS样式。使用`linear-gradient()`函数来创建渐变色,指定方向为纵向(从上到下),并将起始颜色和结束颜色作为参数传递进去,如下所示:
```html
<template>
<div v-bind:style="{ background: 'linear-gradient(to bottom, ' + gradientStartColor + ', ' + gradientEndColor + ')' }">
<!-- 内容 -->
</div>
</template>
```
这样,`div`元素的背景色就会根据`gradientStartColor`和`gradientEndColor`的值来渐变。
你可以根据需要修改起始颜色和结束颜色的值,来实现不同的渐变效果。
html纵向滚动导航栏,html+css+js实现导航栏滚动渐变效果
要实现一个纵向滚动导航栏,可以使用 HTML、CSS 和 JavaScript 来完成。下面是一些步骤和示例代码,可以帮助你实现这个功能。
1. 首先,我们需要创建一个 HTML 结构来定义导航栏和它的菜单项。可以使用 `<ul>` 和 `<li>` 标签来创建一个有序列表,然后在列表项中添加链接。
```html
<nav>
<ul>
<li><a href="#section1">Section 1</a></li>
<li><a href="#section2">Section 2</a></li>
<li><a href="#section3">Section 3</a></li>
<li><a href="#section4">Section 4</a></li>
</ul>
</nav>
```
2. 接下来,我们需要使用 CSS 来设置导航栏的样式。可以给 `<nav>` 元素设置一个固定的高度和宽度,并设置其 `overflow-y` 属性为 `scroll`,以便在内容溢出时显示滚动条。然后,可以设置 `<li>` 元素的样式,例如背景颜色、字体大小和间距。
```css
nav {
height: 400px;
width: 150px;
overflow-y: scroll;
}
li {
background-color: #f6f6f6;
font-size: 16px;
margin-bottom: 10px;
padding: 10px;
}
```
3. 现在,我们需要使用 JavaScript 来添加一些交互性。我们可以使用 `window.addEventListener()` 方法来监听滚动事件,并在导航栏中高亮显示当前所在的节。
```javascript
window.addEventListener('scroll', function() {
var sections = document.querySelectorAll('section');
var navHeight = document.querySelector('nav').offsetHeight;
var currentSectionIndex = 0;
for (var i = 0; i < sections.length; i++) {
if (sections[i].offsetTop - navHeight <= window.scrollY) {
currentSectionIndex = i;
}
}
var activeLink = document.querySelector('nav li.active');
if (activeLink) {
activeLink.classList.remove('active');
}
var newActiveLink = document.querySelectorAll('nav li')[currentSectionIndex];
newActiveLink.classList.add('active');
});
```
在这段代码中,我们首先获取所有的节,并获取导航栏的高度。然后,我们遍历所有的节,如果某个节的顶部位置小于或等于滚动条的位置加上导航栏的高度,就将当前节的索引设置为 `currentSectionIndex`。最后,我们移除当前激活的菜单项的 `active` 类,然后将新的激活菜单项添加 `active` 类。
4. 最后,我们可以使用 CSS 来设置渐变效果。我们可以给 `.active` 类添加一个渐变背景色,使其从浅色逐渐变成深色。这样,用户就可以很容易地看出当前所在的节。
```css
li.active {
background: linear-gradient(to bottom, #f6f6f6, #ccc);
}
```
这样,我们就完成了一个纵向滚动导航栏,并添加了滚动渐变效果。完整的 HTML、CSS 和 JavaScript 代码如下所示:
```html
<!DOCTYPE html>
<html>
<head>
<style>
nav {
height: 400px;
width: 150px;
overflow-y: scroll;
}
li {
background-color: #f6f6f6;
font-size: 16px;
margin-bottom: 10px;
padding: 10px;
}
li.active {
background: linear-gradient(to bottom, #f6f6f6, #ccc);
}
</style>
</head>
<body>
<nav>
<ul>
<li><a href="#section1">Section 1</a></li>
<li><a href="#section2">Section 2</a></li>
<li><a href="#section3">Section 3</a></li>
<li><a href="#section4">Section 4</a></li>
</ul>
</nav>
<section id="section1">Section 1</section>
<section id="section2">Section 2</section>
<section id="section3">Section 3</section>
<section id="section4">Section 4</section>
<script>
window.addEventListener('scroll', function() {
var sections = document.querySelectorAll('section');
var navHeight = document.querySelector('nav').offsetHeight;
var currentSectionIndex = 0;
for (var i = 0; i < sections.length; i++) {
if (sections[i].offsetTop - navHeight <= window.scrollY) {
currentSectionIndex = i;
}
}
var activeLink = document.querySelector('nav li.active');
if (activeLink) {
activeLink.classList.remove('active');
}
var newActiveLink = document.querySelectorAll('nav li')[currentSectionIndex];
newActiveLink.classList.add('active');
});
</script>
</body>
</html>
```
相关推荐
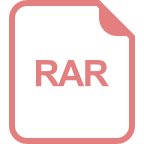
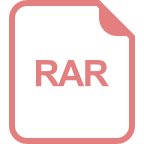
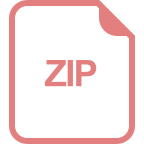





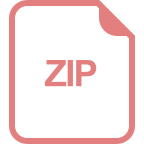
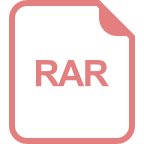
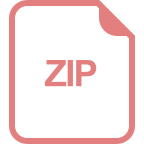
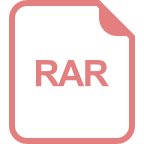
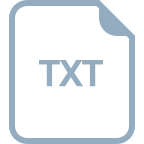
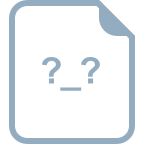
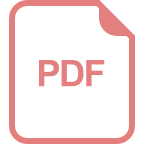
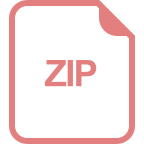
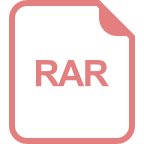