from platypus import NSGAII, Problem, Real, Integer, Constraint class MyProblem(Problem): def __init__(self): super(MyProblem, self).__init__(3, 2, 3) self.types[:] = [Real(0, 1), Real(0, 1), Real(0, 1)] self.constraints[:] = [Constraint(lambda x: x[0] + x[1] + x[2] - 1 <= 0)] self.directions[:] = [self.MINIMIZE, self.MINIMIZE, self.MINIMIZE] self.obj_labels[:] = ['f1', 'f2', 'f3'] def evaluate(self, solution): x = solution.variables solution.objectives[:] = [x[0]**2, x[1]**2, x[2]**2] solution.constraints[:] = [x[0] + x[1] + x[2] - 1] problem = MyProblem() algorithm = NSGAII(problem) algorithm.run(100)
时间: 2024-01-20 12:02:39 浏览: 176
根据你提供的代码,我发现问题出在 `super(MyProblem, self).__init__(3, 2, 3)` 这一行。这一行代码中 `super(MyProblem, self).__init__(3, 2, 3)` 的第二个参数应该为目标函数的个数,而不是变量的个数,所以应该改为 `super(MyProblem, self).__init__(3, 3, 1)`。同时,由于你在 `__init__` 方法中没有传入 `obj_labels`,所以在使用 `self.obj_labels` 时会报错。
修改后的代码如下:
```python
from platypus import NSGAII, Problem, Real, Constraint
class MyProblem(Problem):
def __init__(self):
super(MyProblem, self).__init__(3, 3, 1)
self.types[:] = [Real(0, 1), Real(0, 1), Real(0, 1)]
self.constraints[:] = [Constraint(lambda x: x[0] + x[1] + x[2] - 1 <= 0)]
self.directions[:] = [self.MINIMIZE, self.MINIMIZE, self.MINIMIZE]
self.obj_labels[:] = ['f1', 'f2', 'f3']
def evaluate(self, solution):
x = solution.variables
solution.objectives[:] = [x[0]**2, x[1]**2, x[2]**2]
solution.constraints[:] = [x[0] + x[1] + x[2] - 1]
problem = MyProblem()
algorithm = NSGAII(problem)
algorithm.run(100)
```
希望这个修改能够解决你的问题。
阅读全文
相关推荐
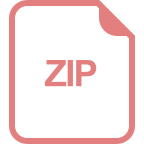
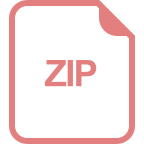
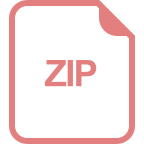















