var img = document.createElement("img"); img.src = src; img.alt = "放大照片"; img.style.maxWidth = "100%"; img.style.maxHeight = "100%"; var scale = 1; // 初始缩放比例 var scaleFactor = 0.1; // 每次滚动的缩放因子 // 添加键盘事件 document.onkeydown = function (e) { // 检查是否显示悬浮窗 if (isPopupVisible) { // 获取所有具有相同类名的图片元素 var imgs = document.querySelectorAll('.preview-image'); var currentIndex = Array.from(imgs).indexOf(img); // 获取当前图片在数组中的索引 switch (e.keyCode) { case 37: // 左箭头键 currentIndex = (currentIndex - 1 + imgs.length) % imgs.length; img.src = imgs[currentIndex].src; break; case 39: // 右箭头键 currentIndex = (currentIndex + 1) % imgs.length; img.src = imgs[currentIndex].src; break; } // 禁用页面滚动条 document.body.style.overflow = "hidden"; } }; // 关闭悬浮窗时恢复页面滚动条 overlay.onclick = function () { document.body.removeChild(popup); document.body.removeChild(overlay); isPopupVisible = false; // 设置悬浮窗显示状态为false // 恢复页面滚动条 document.body.style.overflow = "auto"; document.documentElement.style.overflow = "auto"; };在这个代码里面在关闭悬浮窗后,还是无法恢复滚动条
时间: 2024-04-17 08:26:59 浏览: 88
非常抱歉,之前的回答仍然有误。对于无法恢复滚动条的问题,可以尝试将`overflow`属性设置在`html`元素上,而不仅仅是`body`元素上。
请更新您的代码如下所示:
```javascript
// 关闭悬浮窗时恢复页面滚动条
overlay.onclick = function () {
document.body.removeChild(popup);
document.body.removeChild(overlay);
isPopupVisible = false; // 设置悬浮窗显示状态为false
// 恢复页面滚动条
document.body.style.overflow = "auto";
document.documentElement.style.overflow = "auto";
};
```
在上述代码中,我们将`document.documentElement.style.overflow`也设置为"auto",以确保滚动条可以正确恢复。
希望这次能解决您的问题!如果还有其他疑问,请随时提问。
相关问题
function previewImage(input) { if (input.files && input.files[0]) { var reader = new FileReader(); reader.onload = function (e) { var img = document.createElement("img"); img.src = e.target.result; img.alt = "照片"; img.onclick = function () { showPopup(img.src); }; // 隐藏原始的input元素 input.style.display = "none"; // 添加图片到input元素的父节点 input.parentNode.appendChild(img); }; reader.readAsDataURL(input.files[0]); } } function showPopup(imageSrc) { var popup = document.createElement("div"); var popupImg = document.createElement("img"); var scale = 1; var isDragging = false; var startX, startY, translateX, translateY;这个代码,我需要在添加图片后,能够点击图片,出现悬浮窗放大图片,并且悬浮窗始终在当前最新位置中心
要实现在添加图片后,点击图片出现悬浮窗并放大图片,且悬浮窗始终在当前最新位置的中心,你可以对 `showPopup` 函数进行一些修改。下面是修改后的代码:
```javascript
function showPopup(imageSrc) {
var popup = document.createElement("div");
var popupImg = document.createElement("img");
var scale = 1;
var isDragging = false;
var startX, startY, translateX, translateY;
popup.className = "popup";
popup.style.display = "flex";
popup.style.alignItems = "center";
popup.style.justifyContent = "center";
popup.onclick = function () {
document.body.removeChild(popup);
};
popupImg.src = imageSrc;
popupImg.className = "popup-image";
popupImg.style.maxWidth = "100%";
popupImg.style.maxHeight = "100%";
popupImg.onmousedown = function (e) {
isDragging = true;
startX = e.clientX;
startY = e.clientY;
translateX = 0;
translateY = 0;
};
popupImg.onmousemove = function (e) {
if (isDragging) {
var deltaX = e.clientX - startX;
var deltaY = e.clientY - startY;
translateX += deltaX;
translateY += deltaY;
startX = e.clientX;
startY = e.clientY;
popupImg.style.transform = `translate(${translateX}px, ${translateY}px) scale(${scale})`;
}
};
popupImg.onmouseup = function () {
isDragging = false;
};
popupImg.onwheel = function (e) {
e.preventDefault();
var delta = Math.max(-1, Math.min(1, e.deltaY));
scale += delta * 0.1;
scale = Math.max(0.1, scale);
popupImg.style.transform = `translate(${translateX}px, ${translateY}px) scale(${scale})`;
};
popup.appendChild(popupImg);
document.body.appendChild(popup);
}
```
在修改后的代码中,我添加了一些 CSS 样式来实现悬浮窗在当前最新位置的中心显示。通过 `popup` 元素的样式设置,我们将其显示方式设置为 `flex`,并使用 `alignItems` 和 `justifyContent` 属性来居中显示图片。这样,无论图片的大小和位置如何改变,悬浮窗都会始终在其最新位置的中心。
希望这个修改满足了你的需求!如果你还有其他问题,请随时提问。
function showPopup(src) { if (isPopupVisible) { return; // 如果悬浮窗已经显示,则直接返回 } isPopupVisible = true; // 标记悬浮窗为显示状态 var popup = document.createElement("div"); popup.style.position = "fixed"; popup.style.top = "50%"; popup.style.left = "50%"; popup.style.transform = "translate(-50%, -50%)"; popup.style.backgroundColor = "rgba(0, 0, 0, 0.8)"; popup.style.zIndex = "9999"; popup.style.width = "75%"; // 设置悬浮窗宽度为页面宽度的75% popup.style.height = "75%"; // 设置悬浮窗高度为页面高度的75% // 使图片在悬浮窗居中 var imgContainer = document.createElement("div"); imgContainer.style.display = "flex"; imgContainer.style.justifyContent = "center"; imgContainer.style.alignItems = "center"; imgContainer.style.width = "100%"; imgContainer.style.height = "100%"; var img = document.createElement("img"); img.src = src; img.alt = "放大照片"; img.style.maxWidth = "100%"; img.style.maxHeight = "100%"; var scale = 1; // 初始缩放比例 var scaleFactor = 0.1; // 每次滚动的缩放因子 var imgContainer = document.createElement("div"); imgContainer.className = "image-container"; imgContainer.style.overflow = "hidden"; imgContainer.style.position = "relative"; var isDragging = false; var startX, startY, startScrollLeft, startScrollTop; // 鼠标按下事件 imgContainer.onmousedown = function (e) { e.preventDefault(); isDragging = true; startX = e.clientX; startY = e.clientY; startScrollLeft = imgContainer.scrollLeft; startScrollTop = imgContainer.scrollTop; }; // 鼠标移动事件 imgContainer.onmousemove = function (e) { if (isDragging) { var moveX = e.clientX - startX; var moveY = e.clientY - startY; imgContainer.scrollLeft = startScrollLeft - moveX; imgContainer.scrollTop = startScrollTop - moveY; } }; // 鼠标释放事件 imgContainer.onmouseup = function (e) { isDragging = false; }; // 鼠标离开事件 imgContainer.onmouseleave = function (e) { isDragging = false; };这对图片处理的其他代码,是否会导致图片跳动
根据你提供的代码,我没有看到会导致图片跳动的明显问题。这部分代码主要是处理图片在悬浮窗中的拖拽和滚动功能,不应该直接导致图片跳动的情况。
但是,图片跳动可能与其他代码或样式有关。请确保以下几点:
1. 确保没有其他代码影响了图片的位置和尺寸。例如,检查是否存在其他与图片相关的样式或脚本可能会导致其位置或尺寸发生变化。
2. 确保没有对图片容器或图片设置了不必要的样式,特别是与定位、宽高等相关的样式。
3. 检查是否存在其他与滚动和拖拽相关的代码,可能会与图片处理代码产生冲突。
如果问题仍然存在,请提供更多相关代码或详细描述你想要实现的效果,以便我能够提供更准确的帮助。
阅读全文
相关推荐
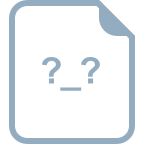
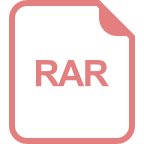
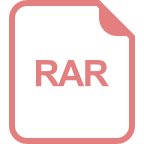





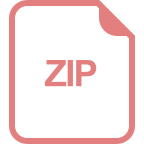
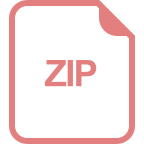
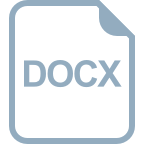
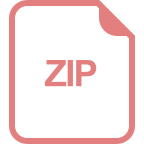
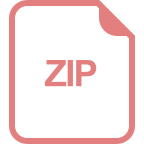
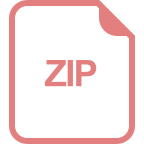