java maplist 转树形
时间: 2023-10-15 19:24:52 浏览: 41
你可以使用递归来将一个具有父子关系的列表转换为树形结构。以下是一个示例代码,演示了如何将一个 `List<Map<String, Object>>` 转换为树形结构:
```java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class MapListToTree {
public static void main(String[] args) {
List<Map<String, Object>> mapList = new ArrayList<>();
Map<String, Object> map1 = new HashMap<>();
map1.put("id", 1);
map1.put("name", "Node 1");
map1.put("parentId", null);
mapList.add(map1);
Map<String, Object> map2 = new HashMap<>();
map2.put("id", 2);
map2.put("name", "Node 2");
map2.put("parentId", 1);
mapList.add(map2);
Map<String, Object> map3 = new HashMap<>();
map3.put("id", 3);
map3.put("name", "Node 3");
map3.put("parentId", null);
mapList.add(map3);
List<Map<String, Object>> tree = buildTree(mapList, null);
System.out.println(tree);
}
private static List<Map<String, Object>> buildTree(List<Map<String, Object>> nodes, Object parentId) {
List<Map<String, Object>> tree = new ArrayList<>();
for (Map<String, Object> node : nodes) {
Object nodeParentId = node.get("parentId");
if ((nodeParentId == null && parentId == null) || (nodeParentId != null && nodeParentId.equals(parentId))) {
List<Map<String, Object>> children = buildTree(nodes, node.get("id"));
node.put("children", children);
tree.add(node);
}
}
return tree;
}
}
```
这段代码首先创建了一个 `mapList`,其中包含了一些具有父子关系的节点。然后,通过调用 `buildTree` 方法,将 `mapList` 转换为树形结构。最后,输出转换后的树形结构。
请根据你的实际情况修改节点的属性和父子关系的表示方式。这只是一个示例,你可以根据需求进行适当的修改。
相关推荐
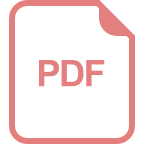
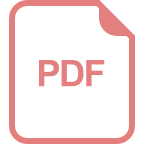














