编写一段c语言代码,这段代码是在Windows的vscode上面运行。 打开串口,向串口发送hex字符串01 03 00 1F 00 01之后接收数据,提取字符串的第四 五位合并成一位并从hex数据翻译成dec数据记录在文档中,这个过程没40ms循环一次
时间: 2024-11-09 11:19:01 浏览: 18
在Windows环境下使用VSCode编写C语言代码,你需要包含相关的头文件,并利用标准库函数如`stdio.h`, `windows.h`, 和 `delay.h`(假设存在`delay.h`用于设置延迟)。以下是一个基本示例:
```c
#include <stdio.h>
#include <windows.h>
#include <string.h>
#include "delay.h" // 假设delay.h提供了一个叫做delay_ms的函数
// 假设你已经设置了正确的串口句柄,这里仅作为占位符
int serialHandle;
void sendHexString(char hexString[], int len) {
for (int i = 0; i < len; i++) {
unsigned char byte = (unsigned char) hexString[i];
printf("Sending %02X\n", byte);
WriteFile(serialHandle, &byte, 1, NULL, NULL); // 发送单个字节
delay_ms(40);
}
}
char extractAndDecode(int data) {
char fourth_fifth[2] = {data >> 4, data & 0x0F};
int combined = ((fourth_fifth[0] << 4) + fourth_fifth[1]);
return combined;
}
void processReceivedData() {
unsigned char buffer[64]; // 假定足够大的接收缓冲区
DWORD bytesRead;
if (ReadFile(serialHandle, buffer, sizeof(buffer), &bytesRead, NULL)) {
for (int i = 0; i < bytesRead / 2; i++) {
int data = (buffer[i*2] << 8) | buffer[i*2+1]; // 解析两个字节为一个16进制数
char decoded = extractAndDecode(data);
printf("Decoded: %d\n", decoded);
}
} else {
printf("Error reading from the serial port.\n");
}
delay_ms(40); // 每次处理完数据后等待40ms
}
int main() {
// 初始化串口和设置波特率...
openSerialPort();
while (true) {
sendHexString("01 03 00 1F 00 01", 6);
processReceivedData();
}
closeSerialPort(); // 关闭串口前记得释放资源
return 0;
}
```
请注意,这只是一个基础示例,实际项目中需要确保串口初始化、错误处理以及可能的串口通信协议都已正确实施。上述代码中`openSerialPort()`和`closeSerialPort()`是未定义的函数,你需要根据你的环境和硬件实现它们。
阅读全文
相关推荐
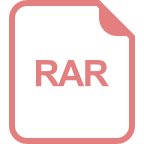
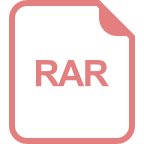
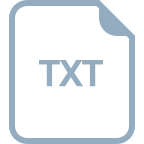
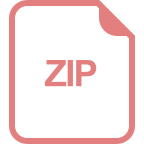
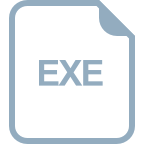







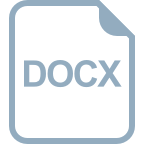
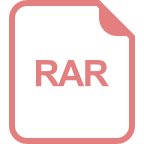
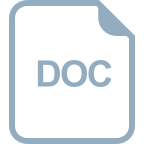


