用unity shader 判断点是否在多边形内
时间: 2023-06-16 15:02:05 浏览: 205
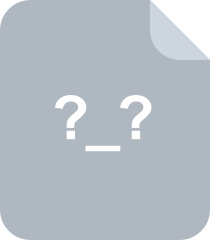
判断点是否多边形内
在Unity中,可以使用ShaderLab编写着色器,但是要在着色器中实现点是否在多边形内比较困难。因此,建议在Unity中使用C#脚本来实现这个功能。
以下是一种实现方法:
1. 首先定义一个多边形类,包含多边形的顶点坐标数组和点是否在多边形内的方法。
```csharp
public class Polygon
{
private Vector2[] vertices;
public Polygon(Vector2[] vertices)
{
this.vertices = vertices;
}
// 判断点是否在多边形内
public bool IsPointInside(Vector2 point)
{
int i, j;
bool c = false;
int nvert = vertices.Length;
for (i = 0, j = nvert - 1; i < nvert; j = i++)
{
if (((vertices[i].y > point.y) != (vertices[j].y > point.y)) &&
(point.x < (vertices[j].x - vertices[i].x) * (point.y - vertices[i].y) / (vertices[j].y - vertices[i].y) + vertices[i].x))
c = !c;
}
return c;
}
}
```
2. 在需要判断点是否在多边形内的脚本中,创建多边形对象,并调用IsPointInside方法判断点是否在多边形内。
```csharp
public class Test : MonoBehaviour
{
public Vector2[] vertices; // 多边形的顶点坐标数组
private Polygon polygon;
void Start()
{
polygon = new Polygon(vertices);
}
void Update()
{
Vector2 point = new Vector2(0.0f, 0.0f); // 需要判断的点的坐标
if (polygon.IsPointInside(point))
{
Debug.Log("点在多边形内");
}
else
{
Debug.Log("点不在多边形内");
}
}
}
```
这样就可以在Unity中判断点是否在多边形内了。需要注意的是,以上方法只适用于凸多边形,对于凹多边形需要进行特殊处理。
阅读全文
相关推荐
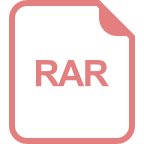
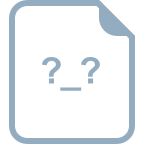
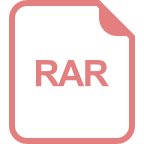
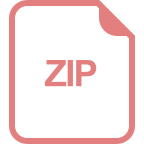
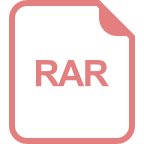
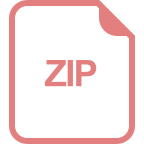
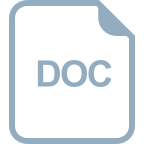
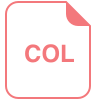





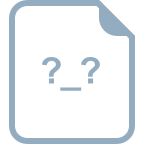
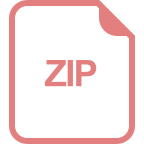