linux c语言读取程序第二行的内容
时间: 2023-12-04 19:02:21 浏览: 132
可以使用C语言中的文件操作函数来读取程序的第二行内容。
以下是一个示例程序,它可以打开当前程序的文件,读取第二行内容并将其输出到标准输出。
```c
#include <stdio.h>
int main() {
FILE *fp;
char line[100];
int count = 1;
fp = fopen(__FILE__, "r");
while (fgets(line, sizeof(line), fp)) {
if (count == 2) {
printf("Second line: %s", line);
break;
}
count++;
}
fclose(fp);
return 0;
}
```
在此示例中,在打开文件后,程序使用 `fgets()` 函数逐行读取文件内容。当计数器为 2 时,程序输出第二行内容并退出循环。
请注意,上述程序使用 `__FILE__` 宏来打开当前程序的文件。如果您想要读取其他程序的文件,请替换该宏为相应的文件路径。
相关问题
linux c语言读取配置文件第二行的内容
读取配置文件可以使用C语言中的文件操作函数来实现。如果要读取配置文件的第二行内容,可以按照以下步骤:
1. 打开文件:使用fopen函数打开文件,并使用文件指针指向该文件。
2. 读取文件:使用fgets函数读取文件的每一行内容,并逐行进行处理。
3. 获取第二行内容:通过循环读取文件中的每一行,使用计数器变量记录当前读取到的行数,当计数器变量为2时,即读取到了第二行,可以获取该行的内容。
4. 关闭文件:使用fclose函数关闭文件,释放资源。
以下是一个示例代码,可以实现读取配置文件第二行内容的功能:
```c
#include <stdio.h>
#define MAX_LINE 1024
int main() {
FILE *file;
char line[MAX_LINE];
int count = 0;
file = fopen("config.txt", "r"); // 打开文件
if (file == NULL) {
printf("Failed to open file.\n");
return 1;
}
while (fgets(line, MAX_LINE, file) != NULL) { // 逐行读取文件
count++;
if (count == 2) { // 获取第二行内容
printf("The second line content is: %s", line);
break;
}
}
fclose(file); // 关闭文件
return 0;
}
```
在上述示例代码中,我们打开了名为config.txt的文件,并使用fgets函数逐行读取文件内容。当计数器变量count为2时,即读取到了第二行,我们就可以获取该行的内容并输出。最后,使用fclose函数关闭文件,释放资源。
linux c语言读取每一行配置文件的信息并保存
可以使用C语言中的文件操作函数来读取配置文件中的每一行信息,并将其保存到一个数据结构中。
以下是一个示例代码,可供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1024
typedef struct {
char key[MAX_LINE_LEN];
char value[MAX_LINE_LEN];
} ConfigEntry;
void parse_config_file(const char* filename, ConfigEntry** entries, size_t* num_entries) {
FILE* fp = fopen(filename, "r");
if (!fp) {
fprintf(stderr, "Error: failed to open config file '%s'\n", filename);
exit(1);
}
ConfigEntry* entries_buf = NULL;
size_t entries_size = 0;
size_t entries_capacity = 0;
char line[MAX_LINE_LEN];
while (fgets(line, MAX_LINE_LEN, fp)) {
// remove trailing newline character
size_t len = strlen(line);
if (len > 0 && line[len-1] == '\n') {
line[len-1] = '\0';
len--;
}
// ignore empty lines and comments
if (len == 0 || line[0] == '#' || line[0] == ';') {
continue;
}
// find the first '=' character
char* eq_pos = strchr(line, '=');
if (!eq_pos) {
fprintf(stderr, "Error: invalid line in config file '%s': '%s'\n", filename, line);
exit(1);
}
// extract key and value
size_t key_len = eq_pos - line;
size_t value_len = len - key_len - 1;
char* key = malloc(key_len+1);
char* value = malloc(value_len+1);
strncpy(key, line, key_len);
key[key_len] = '\0';
strncpy(value, eq_pos+1, value_len);
value[value_len] = '\0';
// add entry to buffer
if (entries_size == entries_capacity) {
entries_capacity = entries_capacity == 0 ? 1 : entries_capacity * 2;
entries_buf = realloc(entries_buf, sizeof(ConfigEntry) * entries_capacity);
}
ConfigEntry* entry = &entries_buf[entries_size++];
strncpy(entry->key, key, MAX_LINE_LEN);
strncpy(entry->value, value, MAX_LINE_LEN);
free(key);
free(value);
}
fclose(fp);
*entries = entries_buf;
*num_entries = entries_size;
}
int main() {
ConfigEntry* entries = NULL;
size_t num_entries = 0;
parse_config_file("config.txt", &entries, &num_entries);
for (size_t i = 0; i < num_entries; i++) {
printf("%s = %s\n", entries[i].key, entries[i].value);
}
free(entries);
return 0;
}
```
在这个示例代码中,我们定义了一个 `ConfigEntry` 结构体,用于保存每一行配置文件中的键值对。我们使用 `fgets()` 函数逐行读取配置文件中的内容,并使用 `strchr()` 函数查找每行字符串中的第一个等号字符,将其前面的部分作为键,后面的部分作为值,存储到 `ConfigEntry` 结构体中。最终,我们将所有的 `ConfigEntry` 结构体保存到一个动态分配的数组中,并输出到控制台上。注意,在实际应用中,我们可能需要对读取到的键值对进行有效性检查和转换等操作。
阅读全文
相关推荐
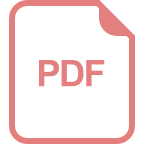
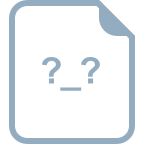
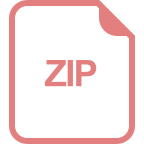


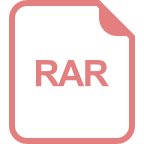
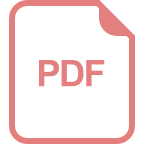
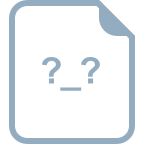
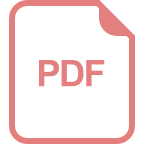
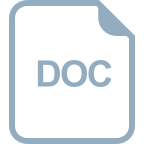
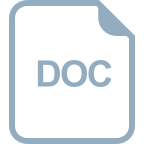
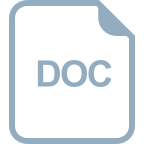




