linux c语言 如何根据进程名来判断进程是否存在
时间: 2024-04-30 15:18:27 浏览: 155
在Linux C语言中,可以使用以下步骤来根据进程名判断进程是否存在:
1. 使用 `opendir()` 函数打开 `/proc` 目录,这个目录下存储了系统中所有进程的运行信息。
2. 遍历 `/proc` 目录下的所有子目录,每个子目录的名称即为对应进程的 PID。
3. 进入每个子目录中,使用 `fopen()` 函数打开 `/proc/[PID]/stat` 文件,该文件中包含了进程的详细信息。
4. 读取 `/proc/[PID]/stat` 文件中的第二个字段,即进程的名称,与要判断的进程名进行比较,如果相等,则说明该进程存在。
5. 关闭文件和目录句柄。
以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
int is_process_exist(const char *proc_name) {
DIR *dir;
struct dirent *entry;
FILE *fp;
char buf[512], name[512];
int pid;
dir = opendir("/proc");
if (!dir) {
perror("opendir failed");
return -1;
}
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type != DT_DIR) {
continue;
}
pid = atoi(entry->d_name);
if (pid == 0) {
continue;
}
snprintf(buf, sizeof(buf), "/proc/%d/stat", pid);
fp = fopen(buf, "r");
if (!fp) {
continue;
}
fgets(buf, sizeof(buf), fp);
sscanf(buf, "%d (%[^)]", &pid, name);
if (strcmp(name, proc_name) == 0) {
fclose(fp);
closedir(dir);
return 1;
}
fclose(fp);
}
closedir(dir);
return 0;
}
```
调用 `is_process_exist()` 函数并传入要判断的进程名,即可返回该进程是否存在的结果。
阅读全文
相关推荐

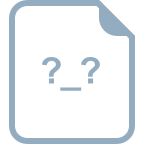
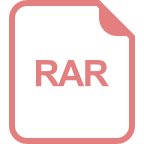
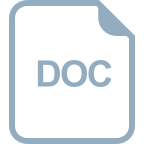
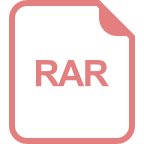
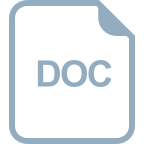
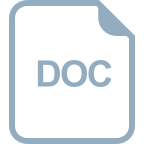
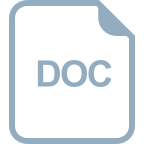
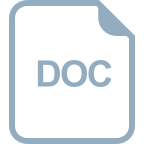
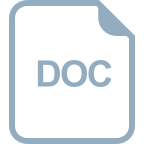
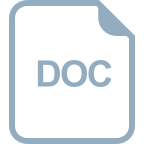




