请在Linux利用c语言写程序,使用共享内存在进程A和进程B之间发送数据,进程A向进程B发送hello,进程B收到进程A的数据hello后发送world给进程A
时间: 2023-08-31 09:43:52 浏览: 85
### 回答1:
在Linux系统中,可以使用共享内存在进程A和进程B之间传递数据。以下是一个用C语言实现的简单示例。
进程A代码:
```
#include <stdio.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <string.h>
#define BUF_SIZE 100
int main()
{
int shmid;
char *shm_addr;
if ((shmid = shmget(IPC_PRIVATE, BUF_SIZE, IPC_CREAT | 0666)) < 0) {
perror("shmget error");
return 1;
}
if ((shm_addr = shmat(shmid, NULL, 0)) == (char *)-1) {
perror("shmat error");
return 1;
}
strcpy(shm_addr, "hello");
printf("进程A向进程B发送数据: %s\n", shm_addr);
if (shmdt(shm_addr) < 0) {
perror("shmdt error");
return 1;
}
return 0;
}
```
进程B代码:
```
#include <stdio.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <string.h>
#define BUF_SIZE 100
int main()
{
int shmid;
char *shm_addr;
if ((shmid = shmget(IPC_PRIVATE, BUF_SIZE, 0666)) < 0) {
perror("shmget error");
return 1;
}
if ((shm_addr = shmat(shmid, NULL, 0)) == (char *)-1) {
perror("shmat error");
return 1;
}
printf("进程B接收到进程A的数据: %s\n", shm_addr);
strcpy(shm_addr, "world");
printf("进程B向进程A发送数据: %s\n", shm_addr);
if (shmdt(shm_addr) < 0) {
perror("shmdt error");
return 1;
}
return 0;
}
```
这两个进程可以分别在不同的终端执行,并在共享内存中传递数据。请注意,共
### 回答2:
在Linux上使用C语言编写程序,可以使用共享内存实现进程A和进程B之间的数据传输。下面是一个简单的示例代码:
进程A:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#define SHM_SIZE 1024
int main() {
key_t key = ftok(".", 'A');
int shmid = shmget(key, SHM_SIZE, IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget");
exit(1);
}
char* shared_memory = (char*)shmat(shmid, NULL, 0);
if (shared_memory == (char*)(-1)) {
perror("shmat");
exit(1);
}
sprintf(shared_memory, "hello");
printf("Process A sends: %s\n", shared_memory);
while (1) {
// 等待进程B写入数据
if (shared_memory[0] == '*') {
printf("Process B sends: %s\n", shared_memory + 1);
break;
}
}
shmdt(shared_memory);
shmctl(shmid, IPC_RMID, NULL);
return 0;
}
```
进程B:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#define SHM_SIZE 1024
int main() {
key_t key = ftok(".", 'A');
int shmid = shmget(key, SHM_SIZE, IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget");
exit(1);
}
char* shared_memory = (char*)shmat(shmid, NULL, 0);
if (shared_memory == (char*)(-1)) {
perror("shmat");
exit(1);
}
while (shared_memory[0] != 'h') {
; // 等待进程A写入数据
}
printf("Process A sends: %s\n", shared_memory);
sprintf(shared_memory, "*world"); // 在数据前加上*标记
shmdt(shared_memory);
return 0;
}
```
以上是一个简单的共享内存通信的例子,进程A向共享内存写入数据"hello",然后进程B从共享内存读取数据,并发送"world"给进程A。进程A通过检查共享内存的首字符来判断是否收到进程B发送的数据。请注意,此示例仅用于演示目的,实际应用中还需要进行错误处理和同步等更复杂的操作。
### 回答3:
要在Linux上使用C语言编写程序,在进程A和进程B之间使用共享内存发送数据,有以下几个步骤:
1. 首先,需要创建一个共享内存段,让进程A和进程B都能够访问。可以使用shmget函数来创建共享内存段,其原型如下:
```
int shmget(key_t key, size_t size, int shmflg);
```
这个函数将返回一个共享内存标识符,可以用来在进程间共享该内存段。
2. 在进程A中,使用shmat函数将共享内存段连接到A进程的地址空间中,使进程A能够访问该内存段。shmat函数的原型如下:
```
void *shmat(int shmid, const void *shmaddr, int shmflg);
```
在连接共享内存后,进程A可以直接访问内存中的数据。
3. 进程A向共享内存写入数据。可以使用strcpy或memcpy等函数将要发送的数据写入共享内存中。
4. 在进程B中,同样使用shmat函数将共享内存段连接到B进程的地址空间中。
5. 进程B从共享内存读取数据,并检查是否收到了来自进程A的数据。如果收到了数据,进程B可以用strcpy或memcpy等函数将要发送给进程A的数据写入共享内存中。
6. 进程B完成数据写入后,可以使用shmdt函数将共享内存段从进程B的地址空间中分离。shmdt函数的原型如下:
```
int shmdt(const void *shmaddr);
```
这样,进程B就不再能够访问该内存段。
7. 在进程A中,同样使用shmdt函数将共享内存段从进程A的地址空间中分离。
8. 最后,可以使用shmctl函数来删除共享内存段。shmctl函数的原型如下:
```
int shmctl(int shmid, int cmd, struct shmid_ds *buf);
```
这样,程序运行结束后,就会清除共享内存。
通过以上步骤,在进程A和进程B之间可以使用共享内存进行数据通信。进程A可以向共享内存写入数据,进程B从共享内存中读取数据并做出响应。
相关推荐
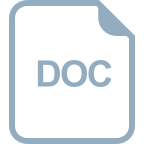
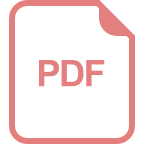
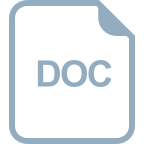










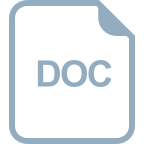
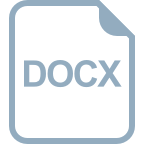
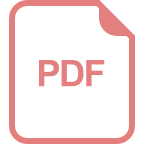
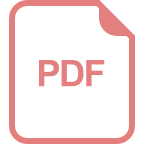
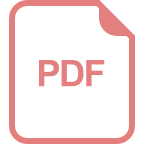