请在Linux利用c语言编写程序,使用共享内存在进程A和进程B之间发送数据,进程A向进程B发送hello,进程B收到进程A的数据hello后发送world给进程A
时间: 2023-09-20 08:05:10 浏览: 110
### 回答1:
在 Linux 中,可以使用共享内存来在进程 A 和进程 B 之间传递数据。
进程 A 中的代码:
```
#include <sys/ipc.h>
#include <sys/shm.h>
#include <string.h>
#include <stdio.h>
int main() {
// 创建共享内存
int shmid = shmget(IPC_PRIVATE, 1024, IPC_CREAT | 0600);
if (shmid < 0) {
perror("shmget");
return 1;
}
// 将共享内存映射到进程的地址空间
char *buf = shmat(shmid, NULL, 0);
if (buf == (char *) -1) {
perror("shmat");
return 1;
}
// 向共享内存写入数据
strcpy(buf, "hello");
// 等待进程 B 读取数据
while (buf[0] != '\0') {
sleep(1);
}
// 打印进程 B 发送的数据
printf("Process B send: %s\n", buf);
// 删除共享内存
if (shmctl(shmid, IPC_RMID, NULL) < 0) {
perror("shmctl");
return 1;
}
return 0;
}
```
进程 B 中的代码:
```
#include <sys/ipc.h>
#include <sys/shm.h>
#include <string.h>
#include <stdio.h>
int main() {
// 获取共享内存
int shmid = shmget(IPC_PRIVATE, 1024, 0600);
if (shmid < 0) {
perror("shmget");
return 1;
}
// 将共享内存映射到进程的地址空间
char *buf = shmat(shmid, NULL, 0);
if (buf == (char *) -1) {
perror("shmat");
return 1;
}
// 等待进程 A 发送数据
while (buf[0] == '\0') {
sleep(1);
}
// 打印进程 A 发送的数据
printf("Process A send: %s\n", buf);
//
### 回答2:
在Linux平台上,可以使用共享内存来实现进程之间的通信。下面是一个示例程序,展示了使用C语言在进程A和进程B之间发送数据的过程。
进程A代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/shm.h>
#define SHM_SIZE 1024 // 共享内存大小
int main() {
key_t key = ftok(".", 'A'); // 生成一个key用于获取共享内存标识符
int shmid;
char *shmaddr;
// 创建共享内存段
shmid = shmget(key, SHM_SIZE, IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget");
exit(1);
}
// 将共享内存连接到当前进程的地址空间
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *)-1) {
perror("shmat");
exit(1);
}
// 向共享内存写入数据
strcpy(shmaddr, "hello");
printf("Process A wrote data: %s\n", shmaddr);
// 等待进程B写入数据
while (strncmp(shmaddr, "world", 5) != 0) {
sleep(1);
}
printf("Process A received data: %s\n", shmaddr);
// 分离共享内存
if (shmdt(shmaddr) == -1) {
perror("shmdt");
exit(1);
}
// 删除共享内存段
if (shmctl(shmid, IPC_RMID, 0) == -1) {
perror("shmctl");
exit(1);
}
return 0;
}
```
进程B代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/shm.h>
#define SHM_SIZE 1024 // 共享内存大小
int main() {
key_t key = ftok(".", 'A'); // 与进程A使用相同的key获取共享内存标识符
int shmid;
char *shmaddr;
// 获取共享内存段
shmid = shmget(key, SHM_SIZE, 0666);
if (shmid == -1) {
perror("shmget");
exit(1);
}
// 将共享内存连接到当前进程的地址空间
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *)-1) {
perror("shmat");
exit(1);
}
// 等待进程A写入数据
while (strncmp(shmaddr, "hello", 5) != 0) {
sleep(1);
}
printf("Process B received data: %s\n", shmaddr);
// 向共享内存写入数据
strcpy(shmaddr, "world");
printf("Process B wrote data: %s\n", shmaddr);
// 分离共享内存
if (shmdt(shmaddr) == -1) {
perror("shmdt");
exit(1);
}
return 0;
}
```
运行上述两个程序,进程A会向共享内存写入数据"hello",进程B会读取共享内存中的数据,并等待进程A写入完成后向共享内存写入数据"world"。最终输出的结果为:
```
Process A wrote data: hello
Process B received data: hello
Process B wrote data: world
Process A received data: world
```
### 回答3:
在Linux下,可以使用共享内存机制实现进程间的数据交流。下面是一个简单的示例代码,使用C语言编写:
进程A的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <string.h>
#define SHM_KEY 1234
#define BUFFER_SIZE 100
int main() {
// 创建共享内存
int shmid = shmget(SHM_KEY, BUFFER_SIZE, IPC_CREAT | 0666);
if (shmid < 0) {
perror("shmget");
exit(1);
}
// 连接共享内存
char *shared_memory = (char *)shmat(shmid, NULL, 0);
if (shared_memory == (char *)-1) {
perror("shmat");
exit(1);
}
// 向共享内存写入数据
strcpy(shared_memory, "hello");
// 通知进程B有数据可读
shared_memory[BUFFER_SIZE] = '1';
// 等待进程B的回复
while (shared_memory[BUFFER_SIZE] != '2') {
usleep(100);
}
// 打印进程B的回复
printf("收到进程B的回复:%s\n", shared_memory);
// 断开与共享内存的连接
shmdt(shared_memory);
// 删除共享内存
shmctl(shmid, IPC_RMID, NULL);
return 0;
}
```
进程B的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <string.h>
#define SHM_KEY 1234
#define BUFFER_SIZE 100
int main() {
// 创建共享内存
int shmid = shmget(SHM_KEY, BUFFER_SIZE, IPC_CREAT | 0666);
if (shmid < 0) {
perror("shmget");
exit(1);
}
// 连接共享内存
char *shared_memory = (char *)shmat(shmid, NULL, 0);
if (shared_memory == (char *)-1) {
perror("shmat");
exit(1);
}
// 等待进程A发送数据
while (shared_memory[BUFFER_SIZE] != '1') {
usleep(100);
}
// 打印进程A发送的数据
printf("收到进程A发送的数据:%s\n", shared_memory);
// 向共享内存写入回复
strcpy(shared_memory, "world");
// 通知进程A已回复完成
shared_memory[BUFFER_SIZE] = '2';
// 断开与共享内存的连接
shmdt(shared_memory);
return 0;
}
```
以上代码创建了一个共享内存区域,进程A将"hello"写入共享内存,并通知进程B有数据可读。进程B等待进程A的通知,收到通知后将共享内存中的数据打印出来,并向共享内存写入"world"作为回复,最后通知进程A回复完成。进程A在收到进程B的回复后,打印出回复的数据。这样就完成了进程A向进程B发送"hello",进程B收到后发送"world"的过程。
阅读全文
相关推荐
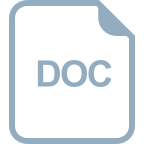
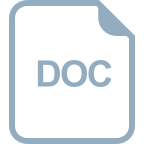
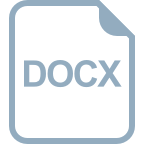









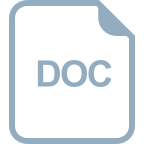
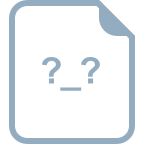
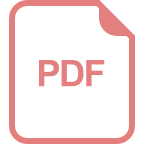
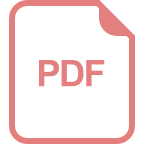
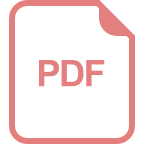
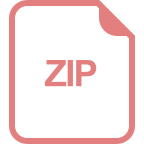
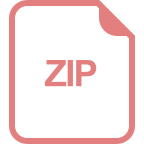
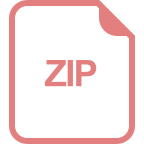