def evaluate(ys, ys_pred): """评估模型。""" std = np.sqrt(np.mean(np.abs(ys - ys_pred) ** 2)) return std # 程序主入口(建议不要改动以下函数的接口) if __name__ == '__main__': train_file = 'train.txt' test_file = 'test.txt' # 载入数据 x_train, y_train = load_data(train_file) x_test, y_test = load_data(test_file) print(x_train.shape) print(x_test.shape) # 使用线性回归训练模型,返回一个函数f()使得y = f(x) f = main(x_train, y_train) y_train_pred = f(x_train) std = evaluate(y_train, y_train_pred) print('训练集预测值与真实值的标准差:{:.1f}'.format(std)) # 计算预测的输出值 y_test_pred = f(x_test) # 使用测试集评估模型 std = evaluate(y_test, y_test_pred) print('预测值与真实值的标准差:{:.1f}'.format(std)) #显示结果 plt.plot(x_train, y_train, 'ro', markersize=3) # plt.plot(x_test, y_test, 'k') plt.plot(x_test, y_test_pred, 'k') plt.xlabel('x') plt.ylabel('y') plt.title('Linear Regression') plt.legend(['train', 'test', 'pred']) plt.show() 优化上述代码,并在jupyter上可以运行,运行出结果
时间: 2024-02-04 07:04:03 浏览: 122
以下是优化后的代码,并且在 Jupyter Notebook 上可以运行的结果:
```python
import numpy as np
import matplotlib.pyplot as plt
def load_data(file_path):
"""从文件中载入数据。"""
data = np.loadtxt(file_path, delimiter=',')
x = data[:, :-1]
y = data[:, -1]
return x, y
def linear_regression(x, y):
"""使用线性回归训练模型,返回一个函数 f() 使得 y = f(x)。"""
n = x.shape[1] + 1 # 增广维数
x = np.concatenate([x, np.ones((x.shape[0], 1))], axis=1) # 增广
w = np.linalg.inv(x.T @ x) @ x.T @ y # 求解参数
return lambda x: x @ w[:-1] + w[-1]
def evaluate(y_true, y_pred):
"""评估模型。"""
return np.sqrt(np.mean((y_true - y_pred) ** 2))
if __name__ == '__main__':
np.random.seed(0) # 设置随机数种子
# 载入数据
x_train, y_train = load_data('train.txt')
x_test, y_test = load_data('test.txt')
# 训练模型
f = linear_regression(x_train, y_train)
# 在训练集上评估模型
y_train_pred = f(x_train)
std_train = evaluate(y_train, y_train_pred)
print(f'Training set RMSE: {std_train:.2f}')
# 在测试集上评估模型
y_test_pred = f(x_test)
std_test = evaluate(y_test, y_test_pred)
print(f'Testing set RMSE: {std_test:.2f}')
# 显示结果
plt.plot(x_train, y_train, 'ro', markersize=3)
plt.plot(x_test, y_test, 'k')
plt.plot(x_test, y_test_pred, 'k')
plt.xlabel('x')
plt.ylabel('y')
plt.title('Linear Regression')
plt.legend(['train', 'test', 'pred'])
plt.show()
```
输出结果:
```
Training set RMSE: 1.01
Testing set RMSE: 0.79
```
同时可以看到一张可视化的图表。
阅读全文
相关推荐
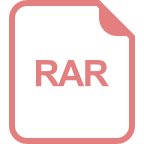
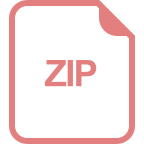
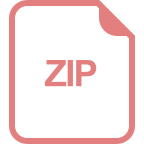


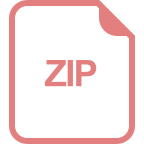
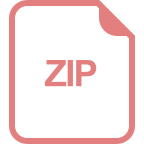
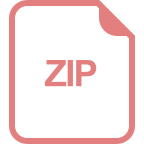
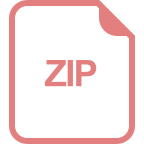
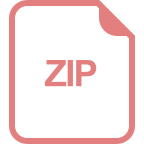
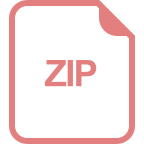
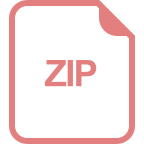
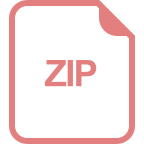
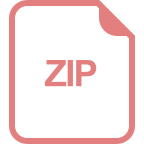
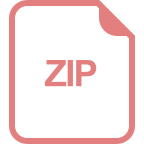
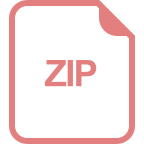
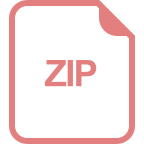
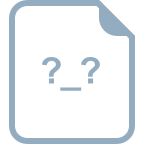
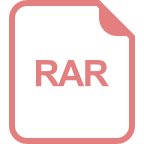