编写程序,根据输入的位序,到已有单链表(含11 22 33 44 55五个元素)中查找相应元素,如找到返回该位序上的元素值,否则返回0。用C语言
时间: 2024-10-11 07:16:44 浏览: 57
在C语言中,你可以通过遍历链表并比较每个节点的值来实现这个功能。首先,你需要定义一个链表结构体,然后创建一个函数来根据位序查找对应的元素。以下是简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
typedef struct Node {
int value;
struct Node* next;
} Node;
// 初始化链表
void createList(Node** head) {
Node* node = (Node*)malloc(sizeof(Node));
node->value = 11;
node->next = NULL;
*head = node;
node = (Node*)malloc(sizeof(Node));
node->value = 22;
node->next = (*head)->next;
(*head)->next = node;
// ... 继续添加其他节点(33, 44, 55)
}
// 检查链表中是否存在对应位序的元素
int findElement(int bitSequence, Node* head) {
while (head != NULL) {
if (bitSequence & 1) { // 如果当前位是1,则检查是否等于node的值
if (head->value == bitSequence) {
return head->value; // 找到了,返回其值
}
} else {
// 当前位是0,跳过此节点
}
bitSequence >>= 1; // 将位序右移一位
head = head->next;
}
return 0; // 没有找到,返回0
}
int main() {
Node* head = NULL;
createList(&head);
int bitSequence = 111; // 用户输入的位序
int result = findElement(bitSequence, head);
if (result != 0) {
printf("找到的元素值: %d\n", result);
} else {
printf("未找到匹配的元素,返回0\n");
}
return 0;
}
```
在这个例子中,`findElement`函数会从头节点开始,逐个检查节点的值是否与给定的位序匹配。如果匹配,就返回该值;如果不匹配,继续检查下一个节点,直到遍历完整个链表。如果没有找到匹配项,最后返回0。
阅读全文
相关推荐
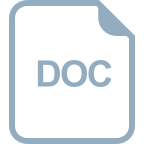
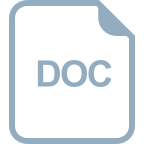
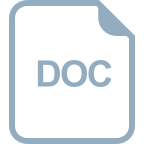
















