定义一个图书类Book,数据成员包含name(书名)、author(作者)、price(定价),为该类定义构造函数,并提供各数据成员的getXXX()和setXXX()函数。在main函数中创建图书对象数组,并将该数组中的元素按图书价格升序排序。
时间: 2023-11-12 07:00:06 浏览: 91
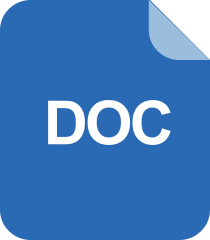
定义一个Book类,实现简单操作实验报告

下面是一个示例代码:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
class Book {
public:
Book(string name, string author, double price) {
this->name = name;
this->author = author;
this->price = price;
}
string getName() const {
return name;
}
void setName(string name) {
this->name = name;
}
string getAuthor() const {
return author;
}
void setAuthor(string author) {
this->author = author;
}
double getPrice() const {
return price;
}
void setPrice(double price) {
this->price = price;
}
private:
string name;
string author;
double price;
};
bool cmp(const Book& a, const Book& b) {
return a.getPrice() < b.getPrice();
}
int main() {
Book books[3] = {
Book("C++ Primer", "Lippman", 89.99),
Book("Thinking in Java", "Eckel", 78.99),
Book("Effective C++", "Meyers", 95.99)
};
sort(books, books + 3, cmp);
for (int i = 0; i < 3; i++) {
cout << books[i].getName() << " " << books[i].getAuthor() << " " << books[i].getPrice() << endl;
}
return 0;
}
```
这个程序定义了一个`Book`类,包含了书名、作者和价格三个数据成员,以及一个构造函数和各个数据成员的getXXX()和setXXX()函数。在`main`函数中,创建了一个包含3本书的数组,并使用`std::sort`函数按照价格升序排序。排序的依据是一个名为`cmp`的比较函数,它比较两个`Book`对象的价格大小。最后,程序输出排序后的书籍信息。
阅读全文
相关推荐
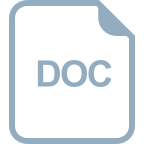














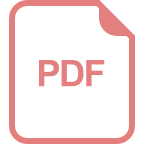
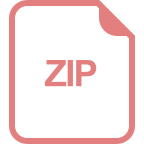