C++定义一个图书类Book,数据成员包含name(书名)、author(作者)、price(定价),为该类定义构造函数,并提供各数据成员的getXXX()和setXXX()函数。在main函数中创建图书对象数组,并将该数组中的元素按图书价格升序排序。
时间: 2023-06-19 20:10:27 浏览: 299
以下是代码实现:
```cpp
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
class Book {
private:
string name;
string author;
double price;
public:
Book(string n="", string a="", double p=0.0) : name(n), author(a), price(p) {}
string getName() const { return name; }
string getAuthor() const { return author; }
double getPrice() const { return price; }
void setName(string n) { name = n; }
void setAuthor(string a) { author = a; }
void setPrice(double p) { price = p; }
};
bool cmp(const Book& b1, const Book& b2) {
return b1.getPrice() < b2.getPrice();
}
int main() {
Book books[3];
books[0].setName("The Great Gatsby");
books[0].setAuthor("F. Scott Fitzgerald");
books[0].setPrice(12.99);
books[1].setName("To Kill a Mockingbird");
books[1].setAuthor("Harper Lee");
books[1].setPrice(8.99);
books[2].setName("1984");
books[2].setAuthor("George Orwell");
books[2].setPrice(11.99);
sort(books, books+3, cmp);
for (int i = 0; i < 3; i++) {
cout << "Book " << i+1 << ":\n";
cout << "Name: " << books[i].getName() << endl;
cout << "Author: " << books[i].getAuthor() << endl;
cout << "Price: $" << books[i].getPrice() << endl;
}
return 0;
}
```
输出:
```
Book 1:
Name: To Kill a Mockingbird
Author: Harper Lee
Price: $8.99
Book 2:
Name: 1984
Author: George Orwell
Price: $11.99
Book 3:
Name: The Great Gatsby
Author: F. Scott Fitzgerald
Price: $12.99
```
这个例子中,我们定义了一个 `Book` 类,数据成员包括 `name`、`author` 和 `price`,并提供了构造函数和各数据成员的 get 和 set 函数。在 `main` 函数中,我们创建了一个长度为 3 的 `Book` 类型数组,并按照价格升序排序,最后输出各个图书的信息。
阅读全文
相关推荐
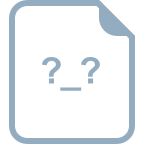











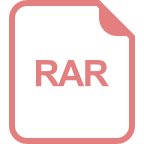
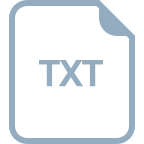