class Book { private: // 图书编号 int book_id; // 书名 string book_name; // 作者 string author; // 出版社 string publisher; // 出版日期 string publish_date; // 价格 double price; 帮我写一份构造方法
时间: 2024-03-04 20:49:30 浏览: 103
好的,根据这个类的成员变量,这里给出一个构造函数的例子:
```c++
class Book {
private:
// 图书编号
int book_id;
// 书名
string book_name;
// 作者
string author;
// 出版社
string publisher;
// 出版日期
string publish_date;
// 价格
double price;
public:
// 构造函数
Book(int id, string name, string author, string publisher, string date, double price) {
book_id = id;
book_name = name;
this->author = author; // 使用this指针来区分成员变量和参数同名的情况
this->publisher = publisher;
publish_date = date;
this->price = price;
}
};
```
这个构造函数包含了6个参数,它们分别对应了类的6个私有成员变量。在函数内部,使用了赋值语句来初始化每一个成员变量。在第10行,使用了this指针来区分成员变量和参数同名的情况。这样,当构造函数被调用时,它会自动将传入的参数赋值给对应的成员变量。
相关问题
list<Book>lst; class Bookstore { private: list<Book> books; public: void WarehousingBook() {//入库书籍 cout << "请输入书名:"; string name; cin >> name; cout << "请输入作者:"; string author; cin >> author; cout << "请输入出版社:"; string publisher; cin >> publisher; cout << "请输入页数:"; int pages; cin >> pages; cout << "请输入最新入库时间(格式:年 月 日):"; int year, month, day; cin >> year >> month >> day; Time latestTime(year, month, day); cout << "请输入进货数量:"; int stock; cin >> stock; cout << "请输入价格:"; double price; cin >> price; list<Book>::iterator it; for (it = lst.begin(); it != lst.end(); it++){ if (it->name == name && it->author == author) { it->stock += stock; cout << "该书库里存在该书,入库成功!" << endl; return; } } Book b(name, author, publisher, pages, latestTime, stock, price); books.push_back(b); cout << "该书库里未存在该书,入库成功!" << endl; } 为什么实现不了
这段代码可能实现不了的原因是因为lst和books是两个不同的list对象,且在函数中只对lst进行了遍历和操作,而将新书籍添加到了books中。所以在函数结束后,books中的内容并没有被更新。如果想要实现添加新书籍的功能,需要将遍历和操作的对象改为books。同时,如果需要在多个函数中共享books这个list对象,需要将其定义为类的私有成员变量。修改后的代码如下所示:
```
class Bookstore {
private:
list<Book> books; // 书籍列表
public:
void WarehousingBook() { // 入库书籍
cout << "请输入书名:";
string name;
cin >> name;
cout << "请输入作者:";
string author;
cin >> author;
cout << "请输入出版社:";
string publisher;
cin >> publisher;
cout << "请输入页数:";
int pages;
cin >> pages;
cout << "请输入最新入库时间(格式:年 月 日):";
int year, month, day;
cin >> year >> month >> day;
Time latestTime(year, month, day);
cout << "请输入进货数量:";
int stock;
cin >> stock;
cout << "请输入价格:";
double price;
cin >> price;
list<Book>::iterator it;
for (it = books.begin(); it != books.end(); it++) {
if (it->name == name && it->author == author) {
it->stock += stock;
cout << "该书库里存在该书,入库成功!" << endl;
return;
}
}
Book b(name, author, publisher, pages, latestTime, stock, price);
books.push_back(b);
cout << "该书库里未存在该书,入库成功!" << endl;
}
};
```
用c++写 问题描述 本图书信息管理系统包括图书的编号、书名、作者、分类号、出版单位、出版时间和价格,可实现新建图书信息管理文件,录入图书信息,查询图书信息,删除图书信息,浏览图书信息。 类的设计: class reader //读者类 class rdatebase //读者库 class book //类图书类 class bdatebase //图书库类 设计要求 (1)用户设置:对软件使用者进行管理,此软件有管理员和普通用户,不同用户有不同的权限及登录密码。管理员用户名: gly,密码: 1234,普通用户用户名:ptyh,密码:4321. (2)用户权限: a.管理员可使用软件内的所有功能,如:新建图书信息管理文件,录入图书信息,查询图书信息,删除图书信息,浏览图书信息。 b.普通用户只能使用部分功能,如: 查询图书信息,测览图书信息,(管理员可看到每本书的价格,但普通用户不能直看)
以下是一个简单的C++图书信息管理系统的实现,包括图书和读者类及其管理库类:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 读者类
class Reader {
public:
Reader(string id, string name) : m_id(id), m_name(name) {}
string get_id() { return m_id; }
string get_name() { return m_name; }
private:
string m_id; // 读者编号
string m_name; // 读者姓名
};
// 读者库类
class ReaderDatabase {
public:
void add_reader(Reader reader) { m_readers.push_back(reader); }
Reader* find_reader(string id) {
for (auto& reader : m_readers) {
if (reader.get_id() == id) {
return &reader;
}
}
return nullptr;
}
private:
vector<Reader> m_readers; // 读者列表
};
// 图书类
class Book {
public:
Book(string id, string title, string author, string category, string publisher, string pub_date, double price)
: m_id(id), m_title(title), m_author(author), m_category(category), m_publisher(publisher), m_pub_date(pub_date), m_price(price) {}
string get_id() { return m_id; }
string get_title() { return m_title; }
string get_author() { return m_author; }
string get_category() { return m_category; }
string get_publisher() { return m_publisher; }
string get_pub_date() { return m_pub_date; }
double get_price() { return m_price; }
private:
string m_id; // 图书编号
string m_title; // 书名
string m_author; // 作者
string m_category; // 分类号
string m_publisher; // 出版单位
string m_pub_date; // 出版时间
double m_price; // 价格
};
// 图书库类
class BookDatabase {
public:
void add_book(Book book) { m_books.push_back(book); }
Book* find_book(string id) {
for (auto& book : m_books) {
if (book.get_id() == id) {
return &book;
}
}
return nullptr;
}
void remove_book(string id) {
for (auto it = m_books.begin(); it != m_books.end(); ++it) {
if (it->get_id() == id) {
m_books.erase(it);
break;
}
}
}
void display_books() {
for (auto& book : m_books) {
cout << "ID: " << book.get_id() << endl;
cout << "Title: " << book.get_title() << endl;
cout << "Author: " << book.get_author() << endl;
cout << "Category: " << book.get_category() << endl;
cout << "Publisher: " << book.get_publisher() << endl;
cout << "Publication Date: " << book.get_pub_date() << endl;
// 只有管理员可以看到价格
if (m_is_admin) {
cout << "Price: " << book.get_price() << endl;
}
cout << endl;
}
}
void set_admin(bool is_admin) { m_is_admin = is_admin; }
private:
vector<Book> m_books; // 图书列表
bool m_is_admin = false; // 是否是管理员
};
int main() {
// 初始化读者库和图书库
ReaderDatabase reader_db;
BookDatabase book_db;
// 添加读者
Reader reader("1001", "张三");
reader_db.add_reader(reader);
// 添加图书
Book book1("B001", "C++ Primer", "Lippman", "TP312C", "机械工业出版社", "2010-08-01", 98.0);
book_db.add_book(book1);
Book book2("B002", "Effective C++", "Scott Meyers", "TP312C", "机械工业出版社", "2005-01-01", 54.0);
book_db.add_book(book2);
// 登录
string username, password;
cout << "Username: ";
cin >> username;
cout << "Password: ";
cin >> password;
// 判断是否是管理员
bool is_admin = (username == "gly" && password == "1234");
// 设置图书库的管理员权限
book_db.set_admin(is_admin);
// 菜单
int choice = 0;
while (true) {
cout << "1. 查询图书信息" << endl;
cout << "2. 浏览图书信息" << endl;
if (is_admin) {
cout << "3. 录入图书信息" << endl;
cout << "4. 删除图书信息" << endl;
}
else {
cout << "(管理员可看到每本书的价格,但普通用户不能直看)" << endl;
}
cout << "0. 退出" << endl;
cout << "Choice: ";
cin >> choice;
if (choice == 0) {
break;
}
else if (choice == 1) {
// 查询图书信息
string id;
cout << "Input Book ID: ";
cin >> id;
Book* book = book_db.find_book(id);
if (book) {
cout << "ID: " << book->get_id() << endl;
cout << "Title: " << book->get_title() << endl;
cout << "Author: " << book->get_author() << endl;
cout << "Category: " << book->get_category() << endl;
cout << "Publisher: " << book->get_publisher() << endl;
cout << "Publication Date: " << book->get_pub_date() << endl;
if (is_admin) {
cout << "Price: " << book->get_price() << endl;
}
}
else {
cout << "Book Not Found!" << endl;
}
}
else if (choice == 2) {
// 浏览图书信息
book_db.display_books();
}
else if (is_admin && choice == 3) {
// 录入图书信息
string id, title, author, category, publisher, pub_date;
double price;
cout << "ID: ";
cin >> id;
cout << "Title: ";
cin >> title;
cout << "Author: ";
cin >> author;
cout << "Category: ";
cin >> category;
cout << "Publisher: ";
cin >> publisher;
cout << "Publication Date: ";
cin >> pub_date;
cout << "Price: ";
cin >> price;
Book book(id, title, author, category, publisher, pub_date, price);
book_db.add_book(book);
}
else if (is_admin && choice == 4) {
// 删除图书信息
string id;
cout << "Input Book ID: ";
cin >> id;
book_db.remove_book(id);
}
else {
cout << "Invalid Choice!" << endl;
}
}
return 0;
}
```
注意,该代码只是一个简单的示例,实际应用中还需要考虑更多的功能和安全性问题。
阅读全文
相关推荐












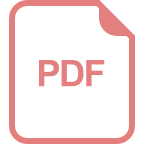
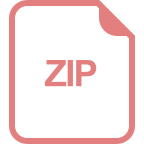
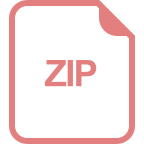
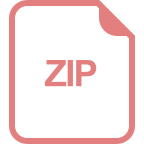