编写一个Book类,用于描述个人藏书,包含作者Author、书名Title、价格Price、出版社Publisher、出版年份Published Year五个属性。然后编写一个测试类BookTest,对Book类进行测试,输出正确的属性值。
时间: 2024-10-17 11:10:46 浏览: 21
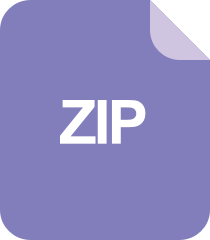
Book_Database:database.exe提供了一个数据库,用于存储有关书籍的信息:书名,作者,出版年份和ISBN。 用户可以添加,更新,删除,搜索和修改条目

当然,首先我们创建一个`Book`类,它具有你提到的属性:
```java
public class Book {
private Author author;
private String title;
private double price;
private String publisher;
private int publishedYear;
// 构造函数
public Book(Author author, String title, double price, String publisher, int publishedYear) {
this.author = author;
this.title = title;
this.price = price;
this.publisher = publisher;
this.publishedYear = publishedYear;
}
// 获取和设置属性的方法
public Author getAuthor() {
return author;
}
public void setAuthor(Author author) {
this.author = author;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public int getPublishedYear() {
return publishedYear;
}
public void setPublishedYear(int publishedYear) {
this.publishedYear = publishedYear;
}
}
```
接下来,我们创建一个`Author`类(这里假设已存在),然后在`BookTest`类中对其进行测试:
```java
import org.junit.Test;
public class BookTest {
@Test
public void testBookProperties() {
// 创建一个Author实例
Author johnDoe = new Author("John Doe", "johndoe@example.com");
// 创建Book实例并设置属性
Book book = new Book(johnDoe, "The Catcher in the Rye", 15.99, "Random House", 1951);
// 测试属性值
System.out.println("Author: " + book.getAuthor().getName());
System.out.println("Title: " + book.getTitle());
System.out.println("Price: $" + book.getPrice());
System.out.println("Publisher: " + book.getPublisher());
System.out.println("Published Year: " + book.getPublishedYear());
// 这里可以添加更多的测试用例,比如检查getter是否正确返回默认值等
}
}
class Author {
private String name;
private String email;
// ...省略构造函数和其他getters和setters
}
```
这个例子展示了如何定义一个`Book`类以及如何在一个测试类中初始化并验证其属性。现在你可以运行`BookTest`类来确认所有属性都能正常工作。
阅读全文
相关推荐
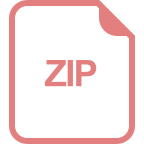
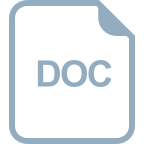






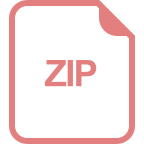
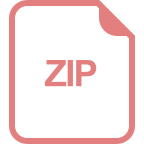
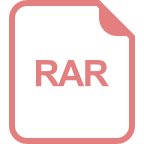