java中、 编写一个Book类,用于描述个人藏书,包含作者Author、书名Title、价格 Price、出版社Publisher、出版年份Published Year共5个属性。然后编写-一个测试类BookTest,对Book类进行测试,输出正确的属性值。
时间: 2024-09-29 14:07:42 浏览: 56
在Java中,你可以首先定义一个`Book`类来描述书籍的基本信息,如下所示:
```java
import java.util.Date; // 导入Date类,假设出版年份需要日期
public class Book {
private String author;
private String title;
private double price;
private String publisher;
private Date publishedYear; // 将出版年份作为Date对象处理
// 构造函数
public Book(String author, String title, double price, String publisher, Date publishedYear) {
this.author = author;
this.title = title;
this.price = price;
this.publisher = publisher;
this.publishedYear = publishedYear;
}
// getter和setter方法
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public Date getPublishedYear() {
return publishedYear;
}
public void setPublishedYear(Date publishedYear) {
this.publishedYear = publishedYear;
}
}
// 测试类BookTest
public class BookTest {
public static void main(String[] args) {
// 创建Book实例
Book book = new Book("J.K.罗琳", "哈利·波特与魔法石", 39.0, "Bloomsbury出版社", new Date(1997, 6, 26));
// 输出属性值
System.out.println("作者: " + book.getAuthor());
System.out.println("书名: " + book.getTitle());
System.out.println("价格: " + book.getPrice());
System.out.println("出版社: " + book.getPublisher());
System.out.println("出版年份: " + book.getPublishedYear());
}
}
```
在`BookTest`的`main`方法中,我们创建了一个新的`Book`对象,并设置了各项属性值,然后通过getter方法打印出各个属性的值。
阅读全文
相关推荐
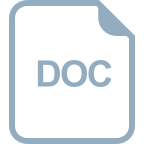
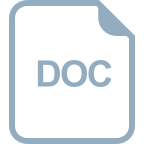
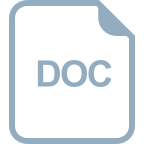







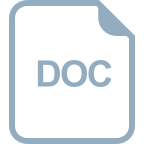
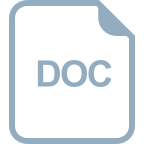
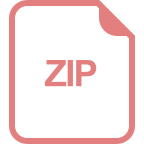
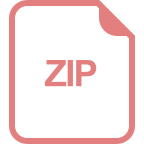
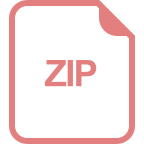
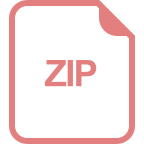
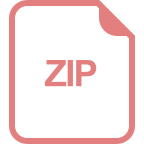