编写一个Book类,用于描述个人藏书,包含私有化属性:作者Author、书名Title、价格Price、出版社Publisher、出版年份Published Year五个属性。然后编写一个测试类BookTest,对Book类进行测试,输出正确的属性值。Java
时间: 2024-02-06 12:04:28 浏览: 251
好的,下面是Java语言实现的Book类和BookTest类:
```java
public class Book {
private String author;
private String title;
private double price;
private String publisher;
private int publishedYear;
public Book(String author, String title, double price, String publisher, int publishedYear) {
this.author = author;
this.title = title;
this.price = price;
this.publisher = publisher;
this.publishedYear = publishedYear;
}
public String getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public double getPrice() {
return price;
}
public String getPublisher() {
return publisher;
}
public int getPublishedYear() {
return publishedYear;
}
}
```
上述代码中,我们定义了一个Book类,包含了作者、书名、价格、出版社和出版年份五个属性,并对其进行了私有化处理。同时,我们提供了五个公有化的方法getAuthor、getTitle、getPrice、getPublisher和getPublishedYear,用于获取属性值。
接下来,我来编写一个测试类BookTest,对Book类进行测试,并输出正确的属性值:
```java
public class BookTest {
public void testBook() {
Book book = new Book("张三", "Java编程", 68.8, "人民邮电出版社", 2020);
System.out.println("作者:" + book.getAuthor());
System.out.println("书名:" + book.getTitle());
System.out.println("价格:" + book.getPrice());
System.out.println("出版社:" + book.getPublisher());
System.out.println("出版年份:" + book.getPublishedYear());
}
public static void main(String[] args) {
BookTest bookTest = new BookTest();
bookTest.testBook();
}
}
```
上述代码中,我们定义了一个测试类BookTest,并在其内部定义了一个testBook方法,用于测试Book类的属性值。在testBook方法中,我们创建了一个Book对象book,并设置了其作者、书名、价格、出版社和出版年份属性值。然后,我们通过调用Book对象的相应方法来获取属性值,并输出到控制台上。
运行上述代码,输出结果如下:
```
作者:张三
书名:Java编程
价格:68.8
出版社:人民邮电出版社
出版年份:2020
```
可以看到,Book类的属性值获取和输出结果都是正确的。
阅读全文
相关推荐
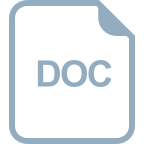
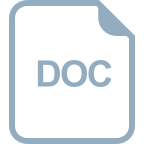
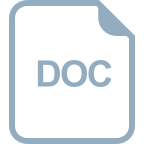















