Book(string name, string author, string publisher, string date, double price, int id) : book_name(name), author(author), publisher(publisher), publish_date(date), price(price), book_id(id) {}这个是什么意思
时间: 2024-03-04 20:50:13 浏览: 110
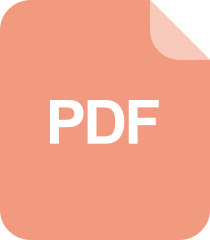
Firebird 开发书记:The_Firebird_Book

这是一个C++中的构造函数,用于创建一个名为Book的类的对象。构造函数包含了六个参数,分别是书名(name)、作者(author)、出版社(publisher)、出版日期(date)、价格(price)、书的编号(id)。在构造函数中,使用了初始化列表来初始化Book类中的成员变量(book_name, author, publisher, publish_date, price和book_id)。这样构造函数被调用时,它会自动将传入的参数赋值给对应的成员变量。
阅读全文
相关推荐
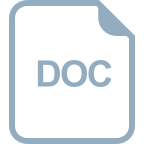
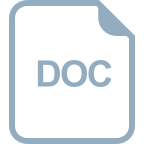












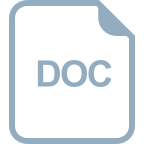
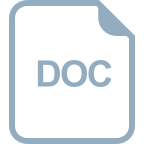
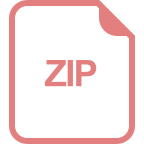