linux w5500代码
时间: 2023-11-21 21:08:40 浏览: 42
以下是使用Linux下的w5500驱动程序的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <fcntl.h>
#include <errno.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <linux/types.h>
#include <linux/spi/spidev.h>
#define SPI_DEVICE "/dev/spidev0.0"
#define W5500_RST 17
uint8_t tx_buf[1024], rx_buf[1024];
int main(int argc, char *argv[]) {
int spi_fd, ret;
struct spi_ioc_transfer xfer;
uint8_t test_cmd[] = {0x90, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00};
uint8_t test_response[128];
spi_fd = open(SPI_DEVICE, O_RDWR);
if (spi_fd < 0) {
printf("Error opening %s: %s\n", SPI_DEVICE, strerror(errno));
return -1;
}
// Set SPI mode
uint8_t mode = SPI_MODE_0;
ret = ioctl(spi_fd, SPI_IOC_WR_MODE, &mode);
if (ret < 0) {
printf("Error setting SPI mode: %s\n", strerror(errno));
return -1;
}
// Set SPI bits per word
uint8_t bits = 8;
ret = ioctl(spi_fd, SPI_IOC_WR_BITS_PER_WORD, &bits);
if (ret < 0) {
printf("Error setting SPI bits per word: %s\n", strerror(errno));
return -1;
}
// Set SPI clock frequency
uint32_t speed = 10000000;
ret = ioctl(spi_fd, SPI_IOC_WR_MAX_SPEED_HZ, &speed);
if (ret < 0) {
printf("Error setting SPI clock frequency: %s\n", strerror(errno));
return -1;
}
// Reset W5500
int gpio_fd = open("/sys/class/gpio/export", O_WRONLY);
if (gpio_fd < 0) {
printf("Error exporting GPIO pin: %s\n", strerror(errno));
return -1;
}
char buf[4];
sprintf(buf, "%d", W5500_RST);
write(gpio_fd, buf, strlen(buf));
close(gpio_fd);
gpio_fd = open("/sys/class/gpio/gpio17/direction", O_WRONLY);
if (gpio_fd < 0) {
printf("Error setting GPIO direction: %s\n", strerror(errno));
return -1;
}
write(gpio_fd, "out", 3);
close(gpio_fd);
gpio_fd = open("/sys/class/gpio/gpio17/value", O_WRONLY);
if (gpio_fd < 0) {
printf("Error setting GPIO value: %s\n", strerror(errno));
return -1;
}
write(gpio_fd, "0", 1);
usleep(1000);
write(gpio_fd, "1", 1);
close(gpio_fd);
// Wait for W5500 to initialize
usleep(1000000);
// Test SPI communication with W5500
memset(&xfer, 0, sizeof(xfer));
xfer.tx_buf = (unsigned long)test_cmd;
xfer.rx_buf = (unsigned long)test_response;
xfer.len = sizeof(test_cmd);
xfer.speed_hz = speed;
xfer.bits_per_word = bits;
ret = ioctl(spi_fd, SPI_IOC_MESSAGE(1), &xfer);
if (ret < 0) {
printf("Error sending SPI command: %s\n", strerror(errno));
return -1;
}
close(spi_fd);
printf("SPI test response: %02X%02X%02X%02X%02X%02X\n", test_response[0], test_response[1], test_response[2], test_response[3], test_response[4], test_response[5]);
return 0;
}
```
这个示例代码使用SPI总线与W5500通信,并测试SPI通信是否正常工作。在示例代码中,我们使用了Linux下的SPIDEV驱动程序,并使用ioctl函数来设置SPI模式、位数和时钟频率。在测试SPI通信之前,我们还将W5500芯片进行了重置。
相关推荐
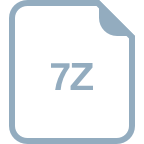














