Linux w5500驱动调用代码
时间: 2023-08-14 17:11:04 浏览: 139
以下是一个使用Linux SPI总线和W5500芯片的驱动程序示例,可供参考:
```c
#include <linux/kernel.h>
#include <linux/module.h>
#include <linux/spi/spi.h>
#include <linux/of_gpio.h>
#include <linux/gpio.h>
#define W5500_SPI_MODE (SPI_MODE_0)
#define W5500_SPI_BITS_PER_WORD (8)
#define W5500_SPI_MAX_SPEED_HZ (10 * 1000 * 1000)
struct w5500_data {
struct spi_device *spi;
struct gpio_desc *reset_gpio;
};
static int w5500_reset(struct w5500_data *w5500)
{
if (!w5500->reset_gpio)
return -ENODEV;
gpiod_set_value_cansleep(w5500->reset_gpio, 1);
msleep(10);
gpiod_set_value_cansleep(w5500->reset_gpio, 0);
msleep(10);
gpiod_set_value_cansleep(w5500->reset_gpio, 1);
msleep(150);
return 0;
}
static int w5500_probe(struct spi_device *spi)
{
struct device *dev = &spi->dev;
struct w5500_data *w5500;
int ret;
w5500 = devm_kzalloc(dev, sizeof(*w5500), GFP_KERNEL);
if (!w5500)
return -ENOMEM;
spi->bits_per_word = W5500_SPI_BITS_PER_WORD;
spi_setup(spi);
w5500->spi = spi;
w5500->reset_gpio = devm_gpiod_get(dev, "reset", GPIOD_OUT_HIGH);
if (IS_ERR(w5500->reset_gpio)) {
ret = PTR_ERR(w5500->reset_gpio);
dev_err(dev, "Failed to get reset GPIO: %d\n", ret);
return ret;
}
ret = w5500_reset(w5500);
if (ret) {
dev_err(dev, "Failed to reset W5500: %d\n", ret);
return ret;
}
return 0;
}
static int w5500_remove(struct spi_device *spi)
{
struct w5500_data *w5500 = spi_get_drvdata(spi);
if (w5500_reset(w5500)) {
dev_warn(&spi->dev, "Failed to reset W5500 on removal\n");
}
return 0;
}
static const struct of_device_id w5500_of_match[] = {
{ .compatible = "wiznet,w5500", },
{ },
};
MODULE_DEVICE_TABLE(of, w5500_of_match);
static struct spi_driver w5500_driver = {
.driver = {
.name = "w5500",
.of_match_table = w5500_of_match,
},
.probe = w5500_probe,
.remove = w5500_remove,
};
module_spi_driver(w5500_driver);
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("W5500 SPI driver");
MODULE_LICENSE("GPL");
```
此驱动程序在设备树中使用以下属性:
```dts
spi {
#address-cells = <1>;
#size-cells = <0>;
w5500@0 {
compatible = "wiznet,w5500";
reg = <0>;
spi-max-frequency = <10000000>;
reset-gpios = <&gpio1 2 GPIO_ACTIVE_HIGH>;
};
};
```
该驱动程序使用 `devm_gpiod_get()` 函数获取重置 GPIO 引脚,以及 `gpiod_set_value_cansleep()` 函数将 GPIO 引脚设置为适当的值。它还使用 SPI API 函数来设置 SPI 总线并进行数据传输。
相关推荐
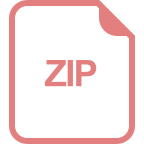
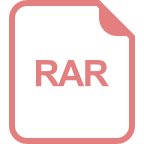














