torch.max()转换为tensorflow代码
时间: 2024-05-13 08:14:46 浏览: 19
在 TensorFlow 中,可以使用 tf.reduce_max() 函数来实现与 torch.max() 相似的功能。
下面是一个示例代码,将 torch.max() 转换为 TensorFlow 代码:
```
import tensorflow as tf
# 定义输入张量 x
x = tf.constant([[1, 2, 3], [4, 5, 6]])
# 使用 tf.reduce_max() 函数实现 torch.max()
max_value = tf.reduce_max(x)
max_value_index = tf.argmax(x)
# 输出结果
print(max_value) # 输出 6
print(max_value_index) # 输出 [1, 2]
```
在上面的示例中,我们首先定义了一个 TensorFlow 常量张量 x。然后,我们使用 tf.reduce_max() 函数来计算张量 x 中的最大值。我们还使用 tf.argmax() 函数来获取最大值的索引。
请注意,这里的 tf.argmax() 函数返回的是一个张量,因此需要使用 .numpy() 方法将其转换为 NumPy 数组或使用 .eval() 方法将其转换为 Python 数值。
相关问题
torch.nn.Module 转换为TensorFlow
将一个 PyTorch 的 nn.Module 转换为 TensorFlow 的代码需要进行一定的手动编写。由于两个框架的底层实现方式不同,因此不能直接转换。以下是一个简单的例子,将一个简单的 PyTorch 模型转换为 TensorFlow 的代码:
```python
import tensorflow as tf
import torch
# 定义 PyTorch 模型
class PyTorchModel(torch.nn.Module):
def __init__(self):
super(PyTorchModel, self).__init__()
self.conv = torch.nn.Conv2d(3, 32, kernel_size=3, stride=1, padding=1)
self.relu = torch.nn.ReLU()
self.pool = torch.nn.MaxPool2d(kernel_size=2, stride=2)
self.fc = torch.nn.Linear(32*8*8, 10)
def forward(self, x):
x = self.conv(x)
x = self.relu(x)
x = self.pool(x)
x = x.view(-1, 32*8*8)
x = self.fc(x)
return x
# 加载 PyTorch 模型
model = PyTorchModel()
model.load_state_dict(torch.load('model.pt'))
# 转换为 TensorFlow 模型
tf_model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, kernel_size=3, strides=1, padding='same', input_shape=(32, 32, 3)),
tf.keras.layers.ReLU(),
tf.keras.layers.MaxPool2D(pool_size=2, strides=2),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(10)
])
# 将 PyTorch 模型的参数赋值给 TensorFlow 模型
for i, layer in enumerate(tf_model.layers):
if hasattr(layer, 'kernel_initializer') and hasattr(layer.kernel_initializer, 'assign'):
layer.kernel_initializer.assign(torch.tensor(model.state_dict()[f'fc.weight'].numpy()))
if hasattr(layer, 'bias_initializer') and hasattr(layer.bias_initializer, 'assign'):
layer.bias_initializer.assign(torch.tensor(model.state_dict()[f'fc.bias'].numpy()))
# 进行预测
x = tf.random.normal((1, 32, 32, 3))
tf_output = tf_model(x)
print(tf_output)
```
需要注意的是,这只是一个简单的例子,实际转换可能会更复杂,需要根据具体的模型进行编写。
将TensorFlow模型转换为pytorch模型
将TensorFlow模型转换为pytorch模型需要进行以下步骤:
1. 导出TensorFlow模型的权重和结构。
2. 创建一个pytorch模型,并且与TensorFlow模型的结构相同。
3. 将TensorFlow模型的权重加载到pytorch模型中。
4. 将pytorch模型保存为.pth文件,以便以后使用。
以下是一个示例代码,展示了如何将TensorFlow模型转换为pytorch模型。
```python
import tensorflow as tf
import torch
# 导出TensorFlow模型的权重和结构
tf_model = tf.keras.models.load_model('tf_model.h5')
tf_model.save_weights('tf_weights.h5')
# 创建一个pytorch模型,与TensorFlow模型的结构相同
pytorch_model = torch.nn.Sequential(
torch.nn.Conv2d(3, 32, kernel_size=3, padding=1),
torch.nn.ReLU(),
torch.nn.MaxPool2d(kernel_size=2),
torch.nn.Conv2d(32, 64, kernel_size=3, padding=1),
torch.nn.ReLU(),
torch.nn.MaxPool2d(kernel_size=2),
torch.nn.Flatten(),
torch.nn.Linear(8 * 8 * 64, 10)
)
# 将TensorFlow模型的权重加载到pytorch模型中
pytorch_model.load_state_dict(torch.load('tf_weights.h5'))
# 将pytorch模型保存为.pth文件
torch.save(pytorch_model.state_dict(), 'pytorch_model.pth')
```
相关推荐
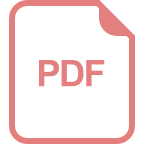
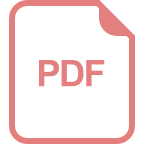
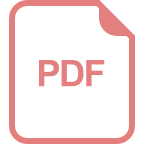












