c++ http上下载bin文件
时间: 2024-03-29 16:37:07 浏览: 14
你可以使用C++的网络库来实现HTTP下载。以下是一个使用libcurl库和C++11的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <curl/curl.h>
// 回调函数,将下载的数据写入文件
static size_t write_callback(char *ptr, size_t size, size_t nmemb, void *userdata) {
std::ofstream *file = reinterpret_cast<std::ofstream*>(userdata);
file->write(ptr, size * nmemb);
return size * nmemb;
}
int main() {
CURL *curl_handle;
CURLcode res;
std::string url = "http://example.com/file.bin"; // 要下载的文件URL
std::string file_path = "/path/to/file.bin"; // 下载后保存的文件路径
std::ofstream file(file_path, std::ios::binary); // 打开二进制文件输出流
curl_global_init(CURL_GLOBAL_DEFAULT);
curl_handle = curl_easy_init();
curl_easy_setopt(curl_handle, CURLOPT_URL, url.c_str());
curl_easy_setopt(curl_handle, CURLOPT_WRITEFUNCTION, write_callback);
curl_easy_setopt(curl_handle, CURLOPT_WRITEDATA, &file);
res = curl_easy_perform(curl_handle);
if(res != CURLE_OK) {
std::cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << std::endl;
}
curl_easy_cleanup(curl_handle);
curl_global_cleanup();
file.close(); // 关闭文件输出流
return 0;
}
```
该示例使用libcurl库来实现HTTP下载,首先打开一个文件输出流并传递给回调函数。在回调函数中,将下载的数据写入文件。然后,使用curl_easy_setopt函数设置要下载的URL、回调函数和回调函数的参数。最后,使用curl_easy_perform函数执行HTTP下载。如果下载成功,文件将保存到指定的路径。
相关推荐
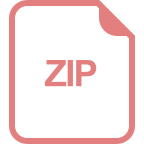
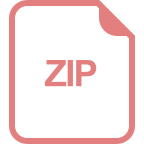














