decoder_lstm2, decoder_bn是什么?自己定义的还是系统提供的?
时间: 2024-03-26 07:37:39 浏览: 64
decoder_lstm2和decoder_bn是在神经网络模型中定义的组件,可能是由开发者自己定义的,也可能是在现有的深度学习框架(例如TensorFlow或PyTorch)中提供的。通常,decoder_lstm2是指解码器中的第二个长短时记忆网络(LSTM),用于将编码器输出的信息转换为生成的文本序列。而decoder_bn是指解码器中的批量归一化层(Batch Normalization),用于加速神经网络的训练并提高模型的性能。这些组件的具体定义和作用可能会因模型而异。
相关问题
class Decoder(nn.Module): def __init__(self,decoder_embedding_num,decoder_hidden_num,ch_corpus_len): super().__init__() self.embedding = nn.Embedding(ch_corpus_len,decoder_embedding_num) self.lstm = nn.LSTM(decoder_embedding_num,decoder_hidden_num,batch_first=True) def forward(self,decoder_input,hidden): embedding = self.embedding(decoder_input) decoder_output,decoder_hidden = self.lstm(embedding,hidden) return decoder_output,decoder_hidden解释每行代码的含义
- 第一行定义了一个名为 Decoder 的类,继承了 nn.Module 类。
- 第二行定义了该类的构造函数,构造函数中有三个参数:decoder_embedding_num 表示解码器嵌入层的维度,decoder_hidden_num 表示解码器 LSTM 层的隐藏层维度,ch_corpus_len 表示中文语料库中字符的数量。
- 第三行调用了 nn.Module 类的构造函数。
- 第四行使用 nn.Embedding 类创建了一个嵌入层对象,该嵌入层的输入维度为 ch_corpus_len,输出维度为 decoder_embedding_num,其中 ch_corpus_len 表示中文语料库中字符的数量。
- 第五行使用 nn.LSTM 类创建了一个 LSTM 层对象,该 LSTM 层的输入维度为 decoder_embedding_num,输出维度为 decoder_hidden_num,batch_first=True 表示输入的第一维是 batch_size。
- 第七至九行定义了 forward 函数,该函数接受两个参数:decoder_input 表示解码器输入序列,hidden 表示解码器的初始隐藏状态。在函数中,首先将 decoder_input 通过嵌入层进行编码,得到编码后的 embedding,接着将 embedding 和 hidden 作为输入传入 LSTM 层中,得到解码器的输出 decoder_output 和新的隐藏状态 decoder_hidden,最后将 decoder_output 和 decoder_hidden 作为输出返回。
# 编码器 def encoder(input_shape, vocab_size, latent_dim): model = tf.keras.models.Sequential([ tf.keras.layers.Embedding(vocab_size, 256, input_shape=input_shape, name="encoder_embedding"), tf.keras.layers.LSTM(latent_dim, name="encode_lstm"), ],name="encoder") return model # 解码器 def decoder(output_shape, vocab_size, latent_dim): model = tf.keras.models.Sequential([ tf.keras.layers.RepeatVector(output_shape[0], input_shape=output_shape, name="decoder_repeatvector"), tf.keras.layers.LSTM(latent_dim, return_sequences=True,name="decode_lstm"), tf.keras.layers.TimeDistributed(tf.keras.layers.Dense(vocab_size, activation='softmax'), name="decoder_td"), ], name="decoder") return model # expected shape=(None, None, 12), found shape=(None, 12, 256) # 定义模型 def build_model(input_shape, output_shape, vocab_size, latent_dim): encoder_model = encoder(input_shape, vocab_size, latent_dim) decoder_model = decoder(output_shape, vocab_size, latent_dim) model = tf.keras.models.Sequential([encoder_model, decoder_model]) return model改正一下模型
根据您提供的代码,您需要将编码器模型的LSTM层的return_sequences参数设置为True,以便正确地将输出形状传递给解码器模型。同时,您还需要将解码器模型的RepeatVector层的输入形状设置为output_shape[1],而不是output_shape[0]。修改后的代码如下所示:
```
# 编码器
def encoder(input_shape, vocab_size, latent_dim):
model = tf.keras.models.Sequential([
tf.keras.layers.Embedding(vocab_size, 256, input_shape=input_shape, name="encoder_embedding"),
tf.keras.layers.LSTM(latent_dim, return_sequences=True, name="encode_lstm"),
], name="encoder")
return model
# 解码器
def decoder(output_shape, vocab_size, latent_dim):
model = tf.keras.models.Sequential([
tf.keras.layers.RepeatVector(output_shape[1], input_shape=(latent_dim,), name="decoder_repeatvector"),
tf.keras.layers.LSTM(latent_dim, return_sequences=True, name="decode_lstm"),
tf.keras.layers.TimeDistributed(tf.keras.layers.Dense(vocab_size, activation='softmax'), name="decoder_td"),
], name="decoder")
return model
# 定义模型
def build_model(input_shape, output_shape, vocab_size, latent_dim):
encoder_model = encoder(input_shape, vocab_size, latent_dim)
decoder_model = decoder(output_shape, vocab_size, latent_dim)
model = tf.keras.models.Sequential([encoder_model, decoder_model])
return model
```
阅读全文
相关推荐










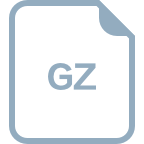



